用Java设计一个简单计算器,具体要求如下:能够实现加减乘除四则运算及开方,乘方运算。运算结果能正常显示,可以清零。可以查看历史记录。使用页面
时间: 2023-11-27 16:54:07 浏览: 129
好的,我可以为您提供一个简单的Java计算器设计代码,使用Java Swing库来创建GUI界面。以下是主要代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class Calculator extends JFrame implements ActionListener {
private JTextField display = new JTextField(20);
private double num1, num2, result;
private String operator;
private String[] history = new String[10];
private int index = 0;
public Calculator() {
super("简单计算器");
JPanel panel1 = new JPanel(new GridLayout(4, 4, 5, 5));
String[] labels = {"7", "8", "9", "+", "4", "5", "6", "-", "1", "2", "3", "*", "0", ".", "=", "/", "√", "x²", "C", "历史记录"};
for (String label : labels) {
JButton button = new JButton(label);
button.setFocusable(false);
button.addActionListener(this);
panel1.add(button);
}
JPanel panel2 = new JPanel(new BorderLayout());
panel2.add(display, BorderLayout.NORTH);
panel2.add(panel1, BorderLayout.CENTER);
JPanel panel3 = new JPanel(new GridLayout(10, 1));
for (int i = 0; i < 10; i++) {
JLabel label = new JLabel();
panel3.add(label);
}
JSplitPane splitPane = new JSplitPane(JSplitPane.HORIZONTAL_SPLIT, panel2, panel3);
splitPane.setDividerLocation(250);
setContentPane(splitPane);
pack();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
}
public void actionPerformed(ActionEvent e) {
String command = e.getActionCommand();
if (command.equals("C")) {
display.setText("");
} else if (command.equals("=")) {
num2 = Double.parseDouble(display.getText());
if (operator.equals("+")) {
result = num1 + num2;
} else if (operator.equals("-")) {
result = num1 - num2;
} else if (operator.equals("*")) {
result = num1 * num2;
} else if (operator.equals("/")) {
result = num1 / num2;
} else if (operator.equals("√")) {
result = Math.sqrt(num1);
} else if (operator.equals("x²")) {
result = Math.pow(num1, 2);
}
display.setText(String.valueOf(result));
String historyItem = num1 + " " + operator + " " + num2 + " = " + result;
history[index % 10] = historyItem;
index++;
} else if (command.equals("历史记录")) {
for (int i = 0; i < 10; i++) {
JLabel label = (JLabel) ((JPanel) getContentPane().getComponent(1)).getComponent(i);
if (history[(index - i - 1) % 10] != null) {
label.setText(history[(index - i - 1) % 10]);
} else {
label.setText("");
}
}
} else {
if (operator == null) {
num1 = Double.parseDouble(display.getText());
} else {
num2 = Double.parseDouble(display.getText());
}
operator = command;
display.setText("");
}
}
public static void main(String[] args) {
new Calculator().setVisible(true);
}
}
```
这个计算器可以实现加减乘除四则运算,开方,乘方以及清零功能。它还记录了最近10次计算历史记录,可以在界面上查看。
阅读全文
相关推荐





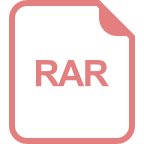












