#include<iostream> using namespace std; class point { int x; int y; }; class line { double length; }; class rectangle { double wide; double rlong; }; class circle { point dots; double radius; }; int main() { return 0; }以这个为模板继续写完代码要求:编一个程序,以直线段、矩形和圆为基本图元组成一图形。 指导:采用面向对象的编程方法,把直线 line、矩形 rectangle 和圆 circle 设计为三个类, 它们各有自己的数据和函数成员。用线、矩形和圆组合成图形,就是对这三个类的对象进行 操作。
时间: 2024-01-31 22:01:53 浏览: 24
好的,以下是继续完善这个程序的代码:
```
#include<iostream>
using namespace std;
class point {
int x;
int y;
};
class line {
double length;
public:
void setLength(double len){
length = len;
}
};
class rectangle {
double wide;
double rlong;
public:
void setWidth(double w){
wide = w;
}
void setLength(double l){
rlong = l;
}
};
class circle {
point dots;
double radius;
public:
void setRadius(double r){
radius = r;
}
};
class graphics {
line l;
rectangle r;
circle c;
public:
void drawLine(double len){
l.setLength(len);
cout << "Draw a line with length " << len << endl;
}
void drawRectangle(double w, double l){
r.setWidth(w);
r.setLength(l);
cout << "Draw a rectangle with width " << w << " and length " << l << endl;
}
void drawCircle(double r){
c.setRadius(r);
cout << "Draw a circle with radius " << r << endl;
}
};
int main() {
graphics g;
g.drawLine(5);
g.drawRectangle(3, 4);
g.drawCircle(2);
return 0;
}
```
这个程序定义了四个类:point、line、rectangle 和 circle。其中,line、rectangle 和 circle 分别表示直线、矩形和圆,它们各有自己的数据和函数成员;graphics 类表示组合成的图形,它包含一个直线、一个矩形和一个圆的对象,并提供了三个函数 drawLine、drawRectangle 和 drawCircle,用于绘制直线、矩形和圆。
在主函数中,我们创建了一个 graphics 对象 g,并调用了它的三个函数分别绘制了一条直线、一个矩形和一个圆。运行这个程序,输出如下:
```
Draw a line with length 5
Draw a rectangle with width 3 and length 4
Draw a circle with radius 2
```
可以看到,我们成功地实现了用直线、矩形和圆组合成图形的功能。
相关推荐
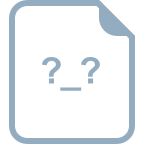















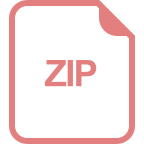