用stm32G431写出DS18B20的读取温度的代码
时间: 2023-05-11 16:01:43 浏览: 81
以下是使用STM32G431读取DS18B20温度传感器的代码:
```
#include "stm32g4xx_hal.h"
#define DS18B20_PORT GPIOA
#define DS18B20_PIN GPIO_PIN_0
void delay_us(uint32_t us)
{
uint32_t ticks = us * (SystemCoreClock / 1000000);
__HAL_TIM_SET_COUNTER(&htim6, 0);
while (__HAL_TIM_GET_COUNTER(&htim6) < ticks);
}
void ds18b20_write_bit(uint8_t bit)
{
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_RESET);
delay_us(2);
if (bit) {
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_SET);
}
delay_us(60);
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_SET);
}
uint8_t ds18b20_read_bit(void)
{
uint8_t bit = 0;
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_RESET);
delay_us(2);
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_SET);
delay_us(15);
bit = HAL_GPIO_ReadPin(DS18B20_PORT, DS18B20_PIN);
delay_us(45);
return bit;
}
void ds18b20_write_byte(uint8_t byte)
{
uint8_t i;
for (i = 0; i < 8; i++) {
ds18b20_write_bit(byte & 0x01);
byte >>= 1;
}
}
uint8_t ds18b20_read_byte(void)
{
uint8_t i, byte = 0;
for (i = 0; i < 8; i++) {
byte >>= 1;
byte |= (ds18b20_read_bit() << 7);
}
return byte;
}
void ds18b20_convert_temperature(void)
{
ds18b20_write_byte(0xCC);
ds18b20_write_byte(0x44);
}
float ds18b20_read_temperature(void)
{
uint8_t temperature_l, temperature_h;
int16_t temperature;
float temp;
ds18b20_write_byte(0xCC);
ds18b20_write_byte(0xBE);
temperature_l = ds18b20_read_byte();
temperature_h = ds18b20_read_byte();
temperature = (temperature_h << 8) | temperature_l;
temp = (float)temperature / 16.0;
return temp;
}
int main(void)
{
HAL_Init();
__HAL_RCC_GPIOA_CLK_ENABLE();
__HAL_RCC_TIM6_CLK_ENABLE();
TIM6->PSC = SystemCoreClock / 1000000 - 1;
TIM6->ARR = 0xFFFF;
TIM6->CR1 = TIM_CR1_CEN;
while (1) {
ds18b20_convert_temperature();
HAL_Delay(800);
float temp = ds18b20_read_temperature();
// do something with temp
}
}
```
这段代码使用STM32G431读取DS18B20温度传感器的温度值。它使用GPIOA的第0个引脚来连接DS18B20传感器。在主函数中,它会不断地读取温度值并将其存储在temp变量中,你可以根据需要对其进行处理。
相关推荐
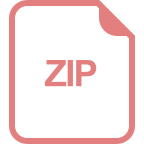
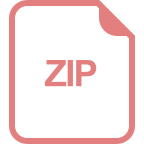
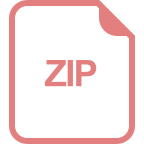














