C++写一个函数给结构体变量初始化
时间: 2024-05-16 16:15:01 浏览: 11
可以使用结构体初始化列表,例如:
```c++
struct Person {
std::string name;
int age;
bool isMale;
};
Person initPerson() {
Person p = {"Tom", 25, true}; // 使用初始化列表初始化结构体变量
return p;
}
```
或者使用构造函数,在构造函数中对结构体成员进行初始化,例如:
```c++
struct Person {
std::string name;
int age;
bool isMale;
Person(std::string name_, int age_, bool isMale_) : name(name_), age(age_), isMale(isMale_) {}
};
Person initPerson() {
Person p("Tom", 25, true); // 调用构造函数初始化结构体变量
return p;
}
```
另外,如果结构体成员是指针类型,需要在初始化时为指针分配内存空间。例如:
```c++
struct Student {
std::string name;
int age;
int *scores;
};
Student initStudent() {
Student s = {"Bob", 18, new int[3]{90, 85, 95}}; // 为指针scores分配内存空间,并初始化数组
return s;
}
```
在使用完指针后,需要手动释放内存空间:
```c++
void freeStudent(Student &s) {
delete[] s.scores; // 释放scores指针指向的数组内存空间
}
```
相关推荐
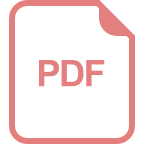
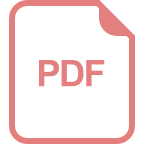
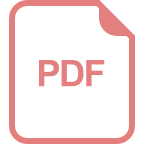














