linux如何使用C调用系统命令
时间: 2023-12-30 21:06:42 浏览: 91
在Linux中,C语言可以使用system()函数来调用系统命令。system()函数的原型如下:
```c
int system(const char *command);
```
其中,command参数是要执行的系统命令,可以是任何有效的shell命令或可执行文件。
例如,如果要在C程序中执行ls命令,可以使用以下代码:
```c
#include <stdio.h>
#include <stdlib.h>
int main()
{
system("ls");
return 0;
}
```
当程序执行到system("ls")时,就会调用系统的shell来执行ls命令,结果会输出到终端上。
需要注意的是,system()函数会阻塞当前进程,直到执行完命令才会返回。如果要在程序中异步执行命令,可以使用fork()和exec()等函数来实现。
相关问题
Linux C语言 程序调用shell命令重启计算机
在Linux环境下,使用C语言程序调用shell命令来重启计算机,你可以通过`system()`函数或者`fork()`, `execve()`系统调用来实现。这里以`system()`函数为例,展示如何操作:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Usage: %s <command>\n", argv[0]);
return -1;
}
// 要执行的shell命令
char* command = "sudo reboot"; // 如果需要密码登录,可以替换为"sudo shutdown -r now"
system(command); // 使用system函数执行shell命令
printf("Restarting the computer...\n");
exit(0);
}
```
在这个例子中,`system(command)`会执行字符串`command`所指定的shell命令。请注意,这涉及到权限问题,如果普通用户直接运行这个程序,可能无法重启或关机。你需要以root用户身份运行,或者提供适当的sudo权限。
Linux C语言 程序调用shell命令关闭计算机
在Linux环境下,使用C语言程序调用shell命令来关闭计算机,通常需要使用`system()`函数或者`popen/pclose`函数组合。这里给出一种简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h> // 包含系统调用相关头文件
int main() {
char *cmd = "sudo shutdown -h now"; // 关闭计算机的命令,"now"表示立即关机
pid_t child_pid = system(cmd); // 使用system函数执行命令
if (child_pid == -1) {
perror("System call failed");
return 1;
} else {
printf("Command executed successfully.\n");
return 0;
}
}
```
在这个例子中,我们首先定义了一个指向shell命令的字符串`cmd`,然后使用`system()`函数执行这个命令。如果成功,`system()`会返回0;如果有错误,它会返回-1,并通过`perror()`函数打印错误信息。
注意:
1. `sudo`用于以管理员权限运行`shutdown`命令,因为关闭计算机通常是管理员操作。
2. `system()`不是安全的,因为它直接执行用户输入的命令,可能存在安全隐患。在实际应用中,推荐使用更安全的方式,如`popen/pclose`、`execlp`等函数。
阅读全文
相关推荐
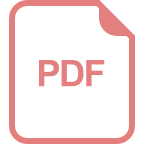
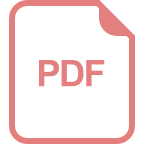
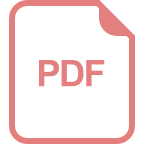
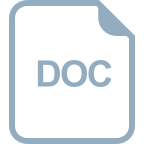
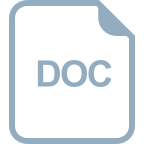
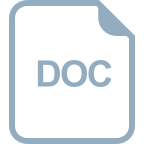




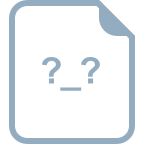
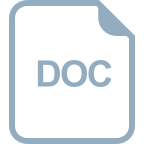
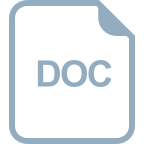



