请使用vue3加setup语法糖 使用element-plus 组件 里面的el-tabe如何可以拖拽改变顺序
时间: 2024-02-27 17:57:26 浏览: 138
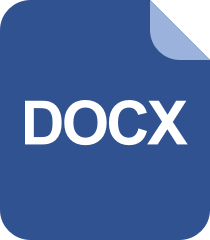
【JavaScript源代码】vue 使用 sortable 实现 el-table 拖拽排序功能.docx
要在Vue3中使用Element Plus的el-table组件并实现拖拽改变顺序,你可以按照以下步骤进行操作:
1. 首先,你需要使用Vue3的Composition API,使用`setup()`函数来初始化组件。
2. 在`setup()`函数中,你需要引入Element Plus的el-table组件和相关的样式。
```javascript
import { ElTable, ElTableColumn } from 'element-plus';
import 'element-plus/lib/theme-chalk/index.css';
```
3. 在`setup()`函数中,你需要定义一个data对象,用于存储el-table的数据和列的定义。这些数据将在el-table中使用。
```javascript
import { ref } from 'vue';
export default {
setup() {
const tableData = ref([
{ name: 'John', age: 25, address: 'New York' },
{ name: 'Jane', age: 20, address: 'London' },
{ name: 'Bob', age: 30, address: 'Paris' },
]);
const tableColumns = ref([
{ prop: 'name', label: 'Name' },
{ prop: 'age', label: 'Age' },
{ prop: 'address', label: 'Address' },
]);
return { tableData, tableColumns };
},
};
```
4. 在`template`标签中,你需要使用el-table组件,并将数据和列绑定到该组件上。
```html
<template>
<el-table :data="tableData" v-loading="loading">
<el-table-column v-for="(column, index) in tableColumns" :key="index" :prop="column.prop" :label="column.label">
</el-table-column>
</el-table>
</template>
```
5. 然后,你需要使用Element Plus的el-table-column组件的`sortable`属性启用列排序功能。此外,你还需要使用`@sort-change`事件来监听列排序的变化,以便更新数据的顺序。
```html
<template>
<el-table :data="tableData" v-loading="loading">
<el-table-column v-for="(column, index) in tableColumns" :key="index" :prop="column.prop" :label="column.label" :sortable="true" @sort-change="handleSortChange">
</el-table-column>
</el-table>
</template>
```
6. 最后,你需要在`methods`中实现`handleSortChange()`方法,该方法将更新数据的顺序。
```javascript
import { ref } from 'vue';
import { ElTable, ElTableColumn } from 'element-plus';
import 'element-plus/lib/theme-chalk/index.css';
export default {
setup() {
const tableData = ref([
{ name: 'John', age: 25, address: 'New York' },
{ name: 'Jane', age: 20, address: 'London' },
{ name: 'Bob', age: 30, address: 'Paris' },
]);
const tableColumns = ref([
{ prop: 'name', label: 'Name', sortable: true },
{ prop: 'age', label: 'Age', sortable: true },
{ prop: 'address', label: 'Address', sortable: true },
]);
const handleSortChange = (params) => {
const { column, prop, order } = params;
tableData.value = tableData.value.sort((a, b) => {
const aVal = a[prop];
const bVal = b[prop];
if (order === 'ascending') {
return aVal > bVal ? 1 : -1;
} else {
return aVal < bVal ? 1 : -1;
}
});
};
return { tableData, tableColumns, handleSortChange };
},
};
```
这样,你就可以在Vue3中使用Element Plus的el-table组件并实现拖拽改变顺序了。
阅读全文
相关推荐
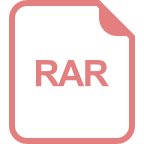



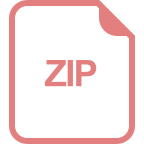
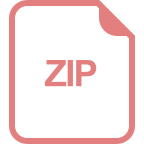
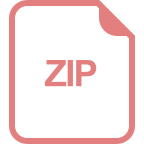
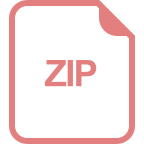
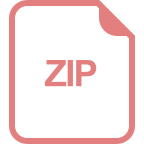
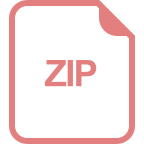
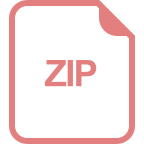
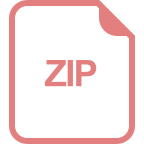
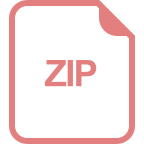
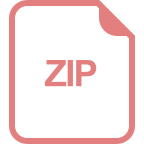
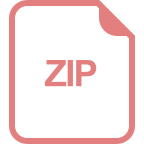
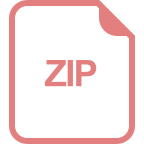
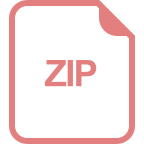