用javaweb写图书管理系统
时间: 2023-06-18 18:03:17 浏览: 111
好的,下面是一个简单的图书管理系统的示例,使用Java Web技术实现。
1. 数据库设计
首先,我们需要设计数据库来存储图书信息。我们可以创建一个名为“library”的数据库,并在其中创建一个名为“books”的表来存储图书信息。该表包含以下字段:
```
id: 图书ID(自增长)
title: 图书标题
author: 作者
publisher: 出版社
price: 价格
```
2. 创建Java Web项目
接下来,我们需要创建一个Java Web项目。您可以使用Eclipse等IDE,或者手动创建项目文件夹和文件。
3. 创建实体类
我们需要创建一个Java类来表示图书对象。这个类应该包含与数据库表中的字段相对应的属性,以及getter和setter方法。
```
public class Book {
private int id;
private String title;
private String author;
private String publisher;
private double price;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getPublisher() {
return publisher;
}
public void setPublisher(String publisher) {
this.publisher = publisher;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
```
4. 创建DAO类
我们需要创建一个DAO(数据访问对象)类,用于连接数据库并实现与图书相关的操作(如添加、删除、更新、查询等)。下面是一个简单的示例:
```
public class BookDAO {
private Connection conn;
public BookDAO() {
try {
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/library";
String user = "root";
String password = "123456";
conn = DriverManager.getConnection(url, user, password);
} catch (Exception e) {
e.printStackTrace();
}
}
public List<Book> getAllBooks() {
List<Book> books = new ArrayList<Book>();
try {
Statement stmt = conn.createStatement();
String sql = "SELECT * FROM books";
ResultSet rs = stmt.executeQuery(sql);
while (rs.next()) {
Book book = new Book();
book.setId(rs.getInt("id"));
book.setTitle(rs.getString("title"));
book.setAuthor(rs.getString("author"));
book.setPublisher(rs.getString("publisher"));
book.setPrice(rs.getDouble("price"));
books.add(book);
}
} catch (Exception e) {
e.printStackTrace();
}
return books;
}
public void addBook(Book book) {
try {
PreparedStatement stmt = conn.prepareStatement("INSERT INTO books(title, author, publisher, price) VALUES (?, ?, ?, ?)");
stmt.setString(1, book.getTitle());
stmt.setString(2, book.getAuthor());
stmt.setString(3, book.getPublisher());
stmt.setDouble(4, book.getPrice());
stmt.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
}
}
public void deleteBook(int id) {
try {
PreparedStatement stmt = conn.prepareStatement("DELETE FROM books WHERE id = ?");
stmt.setInt(1, id);
stmt.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
}
}
public void updateBook(Book book) {
try {
PreparedStatement stmt = conn.prepareStatement("UPDATE books SET title = ?, author = ?, publisher = ?, price = ? WHERE id = ?");
stmt.setString(1, book.getTitle());
stmt.setString(2, book.getAuthor());
stmt.setString(3, book.getPublisher());
stmt.setDouble(4, book.getPrice());
stmt.setInt(5, book.getId());
stmt.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
}
}
public Book getBookById(int id) {
Book book = null;
try {
PreparedStatement stmt = conn.prepareStatement("SELECT * FROM books WHERE id = ?");
stmt.setInt(1, id);
ResultSet rs = stmt.executeQuery();
if (rs.next()) {
book = new Book();
book.setId(rs.getInt("id"));
book.setTitle(rs.getString("title"));
book.setAuthor(rs.getString("author"));
book.setPublisher(rs.getString("publisher"));
book.setPrice(rs.getDouble("price"));
}
} catch (Exception e) {
e.printStackTrace();
}
return book;
}
}
```
5. 创建Servlet类
我们需要创建一个Servlet类来处理HTTP请求,并将请求转发到相应的JSP页面。下面是一个简单的示例:
```
public class BookServlet extends HttpServlet {
private BookDAO bookDAO = new BookDAO();
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String action = request.getServletPath();
switch (action) {
case "/new":
showNewForm(request, response);
break;
case "/insert":
insertBook(request, response);
break;
case "/delete":
deleteBook(request, response);
break;
case "/edit":
showEditForm(request, response);
break;
case "/update":
updateBook(request, response);
break;
default:
listBooks(request, response);
break;
}
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
private void listBooks(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
List<Book> books = bookDAO.getAllBooks();
request.setAttribute("books", books);
RequestDispatcher dispatcher = request.getRequestDispatcher("book-list.jsp");
dispatcher.forward(request, response);
}
private void showNewForm(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
RequestDispatcher dispatcher = request.getRequestDispatcher("book-form.jsp");
dispatcher.forward(request, response);
}
private void insertBook(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String title = request.getParameter("title");
String author = request.getParameter("author");
String publisher = request.getParameter("publisher");
double price = Double.parseDouble(request.getParameter("price"));
Book book = new Book();
book.setTitle(title);
book.setAuthor(author);
book.setPublisher(publisher);
book.setPrice(price);
bookDAO.addBook(book);
response.sendRedirect("list");
}
private void deleteBook(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int id = Integer.parseInt(request.getParameter("id"));
bookDAO.deleteBook(id);
response.sendRedirect("list");
}
private void showEditForm(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int id = Integer.parseInt(request.getParameter("id"));
Book book = bookDAO.getBookById(id);
request.setAttribute("book", book);
RequestDispatcher dispatcher = request.getRequestDispatcher("book-form.jsp");
dispatcher.forward(request, response);
}
private void updateBook(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int id = Integer.parseInt(request.getParameter("id"));
String title = request.getParameter("title");
String author = request.getParameter("author");
String publisher = request.getParameter("publisher");
double price = Double.parseDouble(request.getParameter("price"));
Book book = new Book();
book.setId(id);
book.setTitle(title);
book.setAuthor(author);
book.setPublisher(publisher);
book.setPrice(price);
bookDAO.updateBook(book);
response.sendRedirect("list");
}
}
```
6. 创建JSP页面
最后,我们需要创建JSP页面来显示图书信息和处理用户输入。下面是一个简单的示例:
book-list.jsp
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>图书列表</title>
</head>
<body>
<h1>图书列表</h1>
<table border="1">
<tr>
<th>ID</th>
<th>标题</th>
<th>作者</th>
<th>出版社</th>
<th>价格</th>
<th>操作</th>
</tr>
<c:forEach var="book" items="${books}">
<tr>
<td>${book.id}</td>
<td>${book.title}</td>
<td>${book.author}</td>
<td>${book.publisher}</td>
<td>${book.price}</td>
<td>
<a href="edit?id=${book.id}">编辑</a>
<a href="delete?id=${book.id}" onclick="return confirm('确定要删除该图书吗?')">删除</a>
</td>
</tr>
</c:forEach>
</table>
<br>
<a href="new">添加图书</a>
</body>
</html>
```
book-form.jsp
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>图书管理</title>
</head>
<body>
<h1>图书管理</h1>
<form action="${action}" method="post">
<input type="hidden" name="id" value="${book.id}" />
<label>标题:</label>
<input type="text" name="title" value="${book.title}" /><br>
<label>作者:</label>
<input type="text" name="author" value="${book.author}" /><br>
<label>出版社:</label>
<input type="text" name="publisher" value="${book.publisher}" /><br>
<label>价格:</label>
<input type="text" name="price" value="${book.price}" /><br>
<input type="submit" value="保存" />
</form>
<br>
<a href="list">返回</a>
</body>
</html>
```
7. 配置Web.xml
最后,我们需要在Web.xml文件中配置Servlet。下面是一个简单的示例:
```
<servlet>
<servlet-name>BookServlet</servlet-name>
<servlet-class>com.example.BookServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>BookServlet</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
```
现在,您可以在浏览器中打开http://localhost:8080/来访问该图书管理系统,并进行添加、删除、更新和查询等操作。
阅读全文
相关推荐
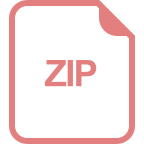
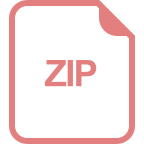
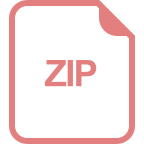














