AttributeError: module 'tensorflow' has no attribute 'Session'. Did you mean: 'version'?
时间: 2023-08-31 08:35:14 浏览: 277
### 回答1:
从TensorFlow 2.0开始,`tf.Session()`已经被弃用了。如果你在使用TensorFlow 2.0及以上版本,需要使用`tf.compat.v1.Session()`来创建会话。
以下是一个修改后的示例代码:
```python
import tensorflow as tf
import numpy as np
# 加载ckpt模型
ckpt_path = 'path/to/your/ckpt/model'
sess = tf.compat.v1.Session()
saver = tf.compat.v1.train.Saver()
saver.restore(sess, ckpt_path)
# 获取模型的输入和输出节点
input_tensor = sess.graph.get_tensor_by_name('input:0')
output_tensor = sess.graph.get_tensor_by_name('output:0')
# 将中文例句转换成模型可以接受的格式
sentence = '这是一段中文文本'
# TODO: 将中文文本转换成数字向量或其他表示方法
input_data = ...
# 运行模型以获取预测结果
feed_dict = {input_tensor: input_data}
probs = sess.run(output_tensor, feed_dict=feed_dict)[0]
# 将概率分布转换为类别标签
label = np.argmax(probs)
print('文本分类结果:', label)
```
需要注意的是,`tf.compat.v1.Session()`创建的会话已经不再支持新版的TensorFlow API。如果你需要使用新版的TensorFlow API,需要使用`tf.compat.v1.enable_eager_execution()`启用Eager Execution模式。
### 回答2:
在TensorFlow 2.0版本中,官方已经放弃了使用Session这个类来建立和运行计算图。相反,TensorFlow 2.0使用了Eager Execution(即即时执行模式),它可以立即执行运算操作,而不需要显式地创建一个会话。
在TensorFlow 1.x版本中,我们必须先创建一个会话对象(Session),然后才能在该会话中运行计算图(Graph)。在1.x版本中,我们可能会看到一些类似于"sess = tf.Session()"这样的代码。然而,在2.0版本中,这些代码会出现AttributeError的错误。
在TensorFlow 2.0中,我们推荐使用Eager Execution模式来代替使用会话(Session)。即时执行模式可以使TensorFlow的运算更加直观和灵活,并且使得调试代码变得容易。我们可以直接运行TensorFlow运算操作(例如张量相乘,卷积等),而不需要先构建计算图和会话。
因此,如果遇到"AttributeError: module 'tensorflow' has no attribute 'Session'"这个错误,原因很可能是您在使用TensorFlow 2.0及以上版本时,尝试使用了Session这个已经过时的类。建议您在迁移代码到TensorFlow 2.0时,修改相关的代码,使用Eager Execution模式来代替Session。
### 回答3:
在TensorFlow 2.x版本中,不再需要使用Session来运行计算图。相反,TensorFlow 2.x默认使用即时执行(Eager Execution)模式,允许立即执行计算操作,无需构建计算图。因此,当出现"AttributeError: module 'tensorflow' has no attribute 'Session'"错误时,可能是因为代码是基于TensorFlow 1.x编写的,而在TensorFlow 2.x中已经不再支持Session。
要解决此问题,可以尝试以下两种方法之一:
1. 更新代码:将代码从TensorFlow 1.x迁移到TensorFlow 2.x,即将使用计算图的部分改为即时执行的方式。
2. 切换TensorFlow版本:如果需要继续使用TensorFlow 1.x的特性,可以安装TensorFlow 1.x版本,然后调用Session来运行计算图。
需要注意的是,TensorFlow 2.x已经成为主推版本,并且提供了更高的性能和易用性。因此,建议尽量迁移到TensorFlow 2.x,并使用即时执行模式,以享受更多的好处。
阅读全文
相关推荐
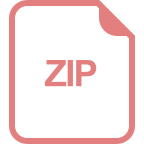
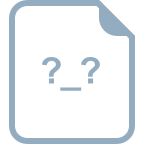







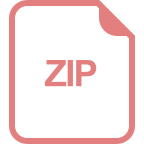
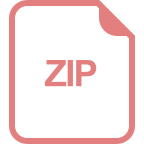
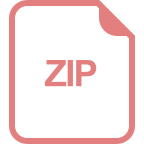
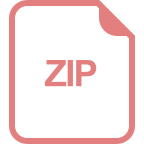
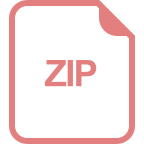