冒泡排序c 语言链表
时间: 2023-06-21 15:09:01 浏览: 152
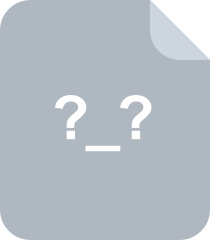
冒泡排序C语言
链表不适合使用冒泡排序算法,因为链表并不是一个连续的数据结构,无法直接交换节点的位置。可以考虑使用其他排序算法,如插入排序、归并排序等。
以下是使用插入排序算法对链表进行排序的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int value;
struct Node *next;
} Node;
void insert(Node **head, int value) {
Node *new_node = (Node *)malloc(sizeof(Node));
new_node->value = value;
new_node->next = NULL;
if (*head == NULL) {
*head = new_node;
} else {
Node *cur_node = *head;
while (cur_node->next != NULL && cur_node->next->value < value) {
cur_node = cur_node->next;
}
new_node->next = cur_node->next;
cur_node->next = new_node;
}
}
void print_list(Node *head) {
Node *cur_node = head;
while (cur_node != NULL) {
printf("%d ", cur_node->value);
cur_node = cur_node->next;
}
printf("\n");
}
void sort_list(Node **head) {
Node *sorted_list = NULL;
Node *cur_node = *head;
while (cur_node != NULL) {
Node *next_node = cur_node->next;
insert(&sorted_list, cur_node->value);
cur_node = next_node;
}
*head = sorted_list;
}
int main() {
Node *head = NULL;
insert(&head, 3);
insert(&head, 1);
insert(&head, 5);
insert(&head, 2);
insert(&head, 4);
printf("Original list: ");
print_list(head);
sort_list(&head);
printf("Sorted list: ");
print_list(head);
return 0;
}
```
输出结果:
```
Original list: 3 1 5 2 4
Sorted list: 1 2 3 4 5
```
阅读全文
相关推荐

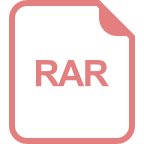



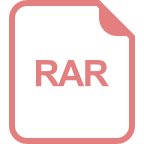
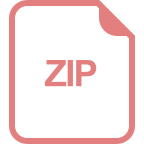
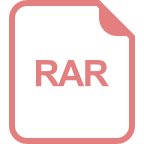
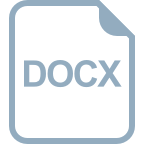
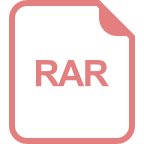
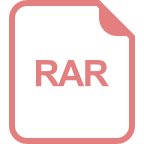
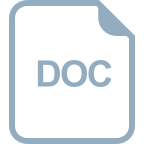