用C语言写链表冒泡排序
时间: 2023-05-24 15:01:22 浏览: 69
以下是一个 C 语言实现链表冒泡排序的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct Node
{
int data;
struct Node *next;
};
// 开始冒泡排序
void bubbleSort(struct Node *start)
{
int swapped, i;
struct Node *ptr1;
struct Node *lptr = NULL;
// 遍历链表节点直到链表的最后一个节点
do
{
swapped = 0;
ptr1 = start;
// 按顺序比较相邻的两个节点值
while (ptr1->next != lptr)
{
if (ptr1->data > ptr1->next->data)
{
int temp = ptr1->data;
ptr1->data = ptr1->next->data;
ptr1->next->data = temp;
swapped = 1;
}
ptr1 = ptr1->next;
}
lptr = ptr1;
}
while (swapped);
}
// 在链表的最后一个节点后面添加新节点
void push(struct Node **head_ref, int new_data)
{
struct Node *new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->data = new_data;
new_node->next = NULL;
struct Node *last = *head_ref;
if (*head_ref == NULL)
{
*head_ref = new_node;
return;
}
while (last->next != NULL)
last = last->next;
last->next = new_node;
return;
}
// 打印链表中的所有元素
void printList(struct Node *node)
{
while (node != NULL)
{
printf("%d ", node->data);
node = node->next;
}
}
// 主函数
int main()
{
struct Node *start = NULL;
push(&start, 5);
push(&start, 20);
push(&start, 4);
push(&start, 3);
push(&start, 30);
printf("链表排序前的元素: \n");
printList(start);
bubbleSort(start);
printf("\n链表排序后的元素: \n");
printList(start);
return 0;
}
```
对于以上代码,我们先定义了一个链表节点结构体,包括一个数据成员和一个指向下一个节点的指针成员。然后编写了冒泡排序和其他帮助函数。在主函数中,我们通过调用 `push()` 函数向链表中添加元素,并在调用 `bubbleSort()` 函数时对链表进行排序。最后再使用 `printList()` 函数打印链表中的所有元素。
希望这个示例代码能够帮助你学习如何使用 C 语言进行链表冒泡排序。
相关推荐
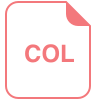
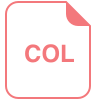
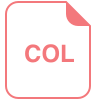
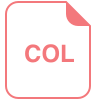
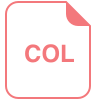









