springboot 文件上传接口
时间: 2024-08-30 08:01:48 浏览: 50
在 Spring Boot 中,创建文件上传接口通常是通过使用 `@PostMapping` 和 `MultipartFile` 来实现的。以下是一个基本的示例:
```java
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile;
@RestController
public class FileUploadController {
@PostMapping("/upload")
public ResponseEntity<String> handleFileUpload(@RequestParam("file") MultipartFile file) {
if (file == null || file.isEmpty()) {
return ResponseEntity.status(HttpStatus.BAD_REQUEST).body("文件不能为空");
}
// 检查文件类型、大小等限制
String fileName = file.getOriginalFilename();
if (!allowedFileTypes.contains(fileName.split("\\.")[1])) {
return ResponseEntity.status(HttpStatus.BAD_REQUEST).body("只允许上传特定类型的文件");
}
if (file.getSize() > maxFileSizeInBytes) {
return ResponseEntity.status(HttpStatus.REQUEST_ENTITY_TOO_LARGE)
.body("文件过大,大小超过 " + maxFileSizeInBytes + " 字节");
}
try {
// 将文件保存到服务器指定位置
String savePath = "/path/to/save/" + fileName;
file.transferTo(new File(savePath));
return ResponseEntity.ok("文件已上传,路径:" + savePath);
} catch (Exception e) {
return ResponseEntity.serverError().body("上传文件时发生错误:" + e.getMessage());
}
}
private List<String> allowedFileTypes; // 需要定义允许上传的文件类型列表
private long maxFileSizeInBytes; // 最大文件大小限制
}
```
这个接口接受一个 `MultipartFile` 参数,并检查文件类型、大小是否符合要求。如果文件上传成功,会在服务器上保存文件并返回上传成功的响应;如果有问题,则返回相应的错误信息。
阅读全文
相关推荐
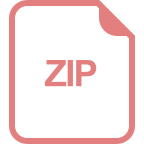
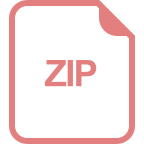

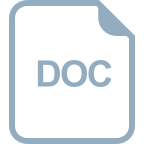


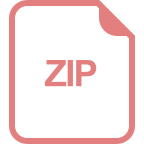
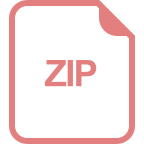







