写一个mybatisplus用redis做二级缓存的代码
时间: 2023-05-25 13:04:52 浏览: 162
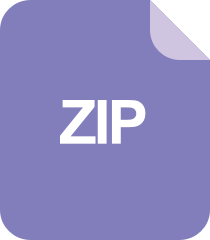
SpringBoot2+MybatisPlus+SpringSecurity+jwt+redis+Vue的前后端分离的商城系统

以下是一个使用MyBatis Plus和Redis作为二级缓存的示例代码:
1. 配置RedisTemplate
```java
@Configuration
public class RedisConfig {
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) {
RedisTemplate<String, Object> redisTemplate = new RedisTemplate<>();
redisTemplate.setConnectionFactory(redisConnectionFactory);
redisTemplate.setKeySerializer(new StringRedisSerializer());
redisTemplate.setValueSerializer(new GenericJackson2JsonRedisSerializer());
redisTemplate.setHashKeySerializer(new StringRedisSerializer());
redisTemplate.setHashValueSerializer(new GenericJackson2JsonRedisSerializer());
return redisTemplate;
}
}
```
2. 配置MyBatis Plus
```java
@Configuration
public class MybatisPlusConfig {
@Bean
public MybatisPlusInterceptor mybatisPlusInterceptor(RedisTemplate<String, Object> redisTemplate) {
MybatisPlusInterceptor interceptor = new MybatisPlusInterceptor();
// 分页插件
interceptor.addInnerInterceptor(new PaginationInnerInterceptor(DbType.MYSQL));
// Redis 二级缓存插件
RedisCache redisCache = new RedisCache(redisTemplate);
Properties properties = new Properties();
properties.setProperty("redisCache.expire", "3600");
properties.setProperty("redisCache.cacheNullObject", "true");
RedisCacheInterceptor redisCacheInterceptor = new RedisCacheInterceptor(redisCache, properties);
interceptor.addInnerInterceptor(redisCacheInterceptor);
return interceptor;
}
@Bean
public ConfigurationCustomizer configurationCustomizer(MybatisPlusInterceptor mybatisPlusInterceptor) {
return configuration -> configuration.addInterceptor(mybatisPlusInterceptor);
}
}
```
3. 使用@CacheNamespace注解开启缓存
```java
@CacheNamespace(implementation = RedisCache.class, eviction = RedisCache.class)
public interface UserMapper extends BaseMapper<User> {
@Cacheable(value = "user:id", key = "#id")
User getUserById(Long id);
@CachePut(value = "user:id", key = "#user.id")
int updateUserById(User user);
@CacheEvict(value = "user:id", key = "#id")
int deleteUserById(Long id);
}
```
在这里,@CacheNamespace注解用于开启Redis缓存,并指定了缓存的实现类和清理策略。
@Cacheable注解用于查询缓存中的数据,@CachePut注解用于更新缓存中的数据,@CacheEvict注解用于删除缓存中的数据。
还可以在@Cacheable注解中指定缓存的名称和缓存的键值,以及在@CachePut和@CacheEvict注解中指定缓存的键值。
注意:在使用Redis作为二级缓存时,需要在mapper中使用@Cacheable注解进行数据的缓存和查询,同时要保证实体类的序列化和反序列化能够正确进行。
阅读全文
相关推荐
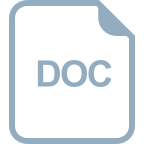
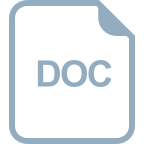





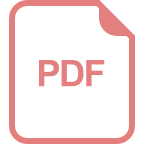
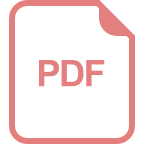

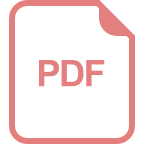


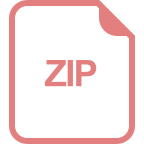
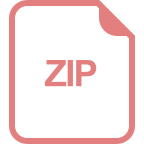
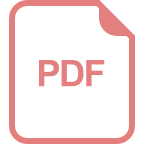
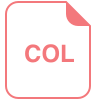
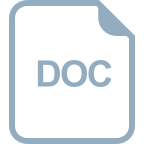