mybatisplus自定义redis二级缓存
时间: 2023-05-26 21:03:14 浏览: 110
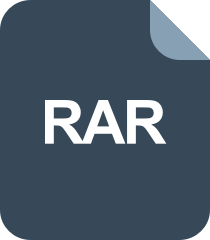
springboot+mybatisplus+redis+spring cache缓存
MybatisPlus自带的二级缓存默认使用了EHCache作为缓存实现,但在一些场景下可能需要使用Redis作为缓存实现。本文将介绍如何在MybatisPlus中自定义Redis二级缓存。
1. 引入Redis相关依赖
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
<version>2.2.2.RELEASE</version>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-pool2</artifactId>
<version>2.6.2</version>
</dependency>
```
2. 自定义RedisCache
```java
public class RedisCache implements Cache {
private String id;
private static RedisTemplate<String, Object> redisTemplate;
public RedisCache(String id) {
this.id = id;
initRedisTemplate();
}
private void initRedisTemplate() {
if (redisTemplate == null) {
synchronized (RedisCache.class) {
if (redisTemplate == null) {
redisTemplate = (RedisTemplate<String, Object>) ApplicationContextHolder.getBean("redisTemplate");
}
}
}
}
@Override
public String getId() {
return id;
}
@Override
public void putObject(Object key, Object value) {
redisTemplate.opsForHash().put(id, key.toString(), value);
}
@Override
public Object getObject(Object key) {
return redisTemplate.opsForHash().get(id, key.toString());
}
@Override
public Object removeObject(Object key) {
redisTemplate.opsForHash().delete(id, key.toString());
return null;
}
@Override
public void clear() {
redisTemplate.delete(id);
}
@Override
public int getSize() {
return redisTemplate.opsForHash().size(id).intValue();
}
@Override
public ReadWriteLock getReadWriteLock() {
return null;
}
}
```
3. 自定义RedisCacheFactory
```java
public class RedisCacheFactory implements CacheFactory {
private static final Map<String, Cache> caches = new ConcurrentHashMap<>(16);
private static RedisTemplate<String, Object> redisTemplate;
public RedisCacheFactory() {
initRedisTemplate();
}
private void initRedisTemplate() {
if (redisTemplate == null) {
synchronized (RedisCacheFactory.class) {
if (redisTemplate == null) {
redisTemplate = (RedisTemplate<String, Object>) ApplicationContextHolder.getBean("redisTemplate");
}
}
}
}
@Override
public Cache getCache(String id) {
Cache cache = caches.get(id);
if (cache == null) {
cache = new RedisCache(id);
caches.put(id, cache);
}
return cache;
}
@Override
public Set<String> getCacheNames() {
return caches.keySet();
}
}
```
4. 配置MybatisPlus
```java
@Configuration
public class MybatisPlusConfig {
@Autowired
private RedisCacheFactory redisCacheFactory;
@Bean
public MybatisPlusInterceptor mybatisPlusInterceptor() throws Exception {
MybatisPlusInterceptor interceptor = new MybatisPlusInterceptor();
Properties props = new Properties();
props.setProperty("cache-enabled", "true");
props.setProperty("local-cache-scope", "STATEMENT");
props.setProperty("local-cache-ref", "local-cache");
// 自定义RedisCacheFactory
props.setProperty("second-level-cache-enabled", "true");
props.setProperty("second-level-cache-type", "org.apache.ibatis.cache.decorators.RedisCache");
interceptor.addInnerInterceptor(new CacheInterceptor(props, redisCacheFactory));
return interceptor;
}
}
```
完成上述步骤后,即可在MybatisPlus中使用自定义的Redis二级缓存。
阅读全文
相关推荐
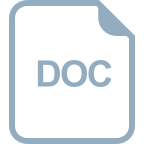
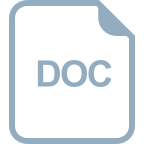



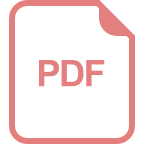
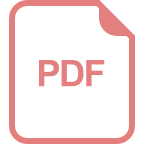
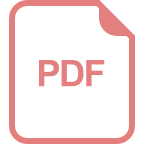
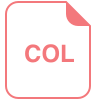

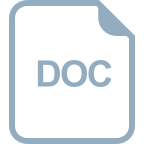
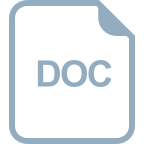
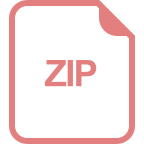
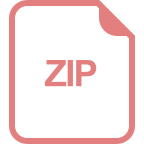
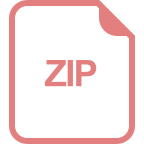
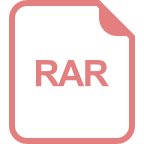
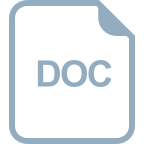
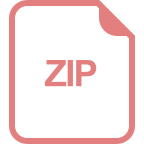