java连接linux主机并读写文件至数据库
时间: 2023-08-14 16:08:25 浏览: 128
可以使用Java中的SSH库,比如JSch,连接到Linux主机,并使用Java中的JDBC库连接到数据库,然后进行读写文件的操作。
以下是一个简单的示例代码,假设需要将Linux主机上的文件/tmp/test.txt中的内容写入到名为test的数据库中的表test_table中:
```java
import com.jcraft.jsch.*;
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.sql.*;
public class Main {
public static void main(String[] args) {
String host = "linux.host.com";
String username = "username";
String password = "password";
String remoteFilePath = "/tmp/test.txt";
String jdbcUrl = "jdbc:mysql://localhost:3306/test";
String jdbcUsername = "root";
String jdbcPassword = "password";
try {
// Connect to Linux host via SSH
JSch jsch = new JSch();
Session session = jsch.getSession(username, host, 22);
session.setPassword(password);
session.setConfig("StrictHostKeyChecking", "no");
session.connect();
Channel channel = session.openChannel("sftp");
channel.connect();
ChannelSftp sftpChannel = (ChannelSftp) channel;
// Read file content from remote file
InputStream inputStream = sftpChannel.get(remoteFilePath);
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(inputStream));
StringBuilder stringBuilder = new StringBuilder();
String line;
while ((line = bufferedReader.readLine()) != null) {
stringBuilder.append(line).append("\n");
}
String fileContent = stringBuilder.toString();
// Write file content to database
Class.forName("com.mysql.cj.jdbc.Driver");
Connection connection = DriverManager.getConnection(jdbcUrl, jdbcUsername, jdbcPassword);
PreparedStatement preparedStatement = connection.prepareStatement("INSERT INTO test_table (content) VALUES (?)");
preparedStatement.setString(1, fileContent);
preparedStatement.executeUpdate();
// Close connections
sftpChannel.disconnect();
session.disconnect();
preparedStatement.close();
connection.close();
} catch (JSchException | SftpException | ClassNotFoundException | SQLException | IllegalAccessException | InstantiationException e) {
e.printStackTrace();
}
}
}
```
注意:这只是一个示例代码,实际情况中需要根据具体的需求进行修改。
阅读全文
相关推荐
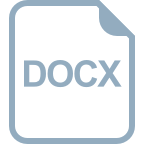
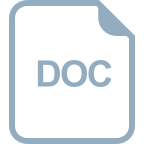
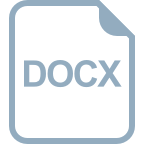
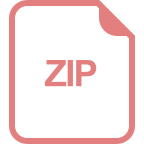
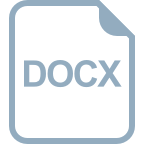
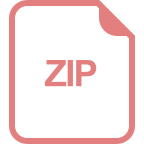
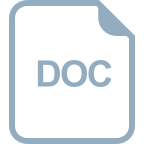
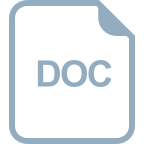
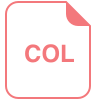
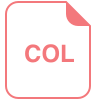
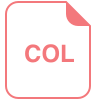
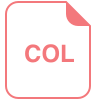
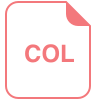
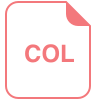
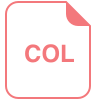