请使用python编写一个对文件内容增删改查的操作
时间: 2024-02-01 19:15:16 浏览: 117
以下是一个简单的对文件内容增删改查的操作示例:
```python
import os
# 定义文件路径
file_path = "test.txt"
# 写入文件内容
def write_file(content):
with open(file_path, "w") as f:
f.write(content)
# 读取文件内容
def read_file():
with open(file_path, "r") as f:
content = f.read()
return content
# 追加文件内容
def append_file(content):
with open(file_path, "a") as f:
f.write(content)
# 修改文件内容
def modify_file(old_str, new_str):
content = read_file()
content = content.replace(old_str, new_str)
write_file(content)
# 删除文件内容
def delete_file(content):
content = read_file()
content = content.replace(content, "")
write_file(content)
# 测试
write_file("hello world\n")
append_file("this is a test\n")
print(read_file())
modify_file("hello", "hi")
print(read_file())
delete_file("this")
print(read_file())
# 删除文件
os.remove(file_path)
```
在该示例中,我们定义了一个文件路径 `file_path`,并提供了五个文件操作函数:
- `write_file(content)`:写入文件内容;
- `read_file()`:读取文件内容;
- `append_file(content)`:追加文件内容;
- `modify_file(old_str, new_str)`:修改文件内容;
- `delete_file(content)`:删除文件内容。
最后,我们对这些函数进行了简单的测试。注意,测试完成后我们还使用 `os.remove()` 函数删除了该文件。
阅读全文
相关推荐
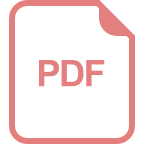
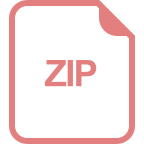
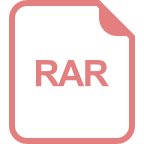















