实现单链表的数据类型 利用.h .c
时间: 2023-06-05 21:02:09 浏览: 56
实现单链表的数据类型需要使用C语言的.h和.c文件。在.h文件中,需要定义单链表的结构体,包括节点的数据域和指针域。同时,声明单链表相关的函数,如新建节点、插入节点、删除节点、查找节点等。在.c文件中,实现上述函数的具体操作,以及其他辅助函数的实现。
下面是一个简单的例子:
## 链表结构体定义
```c
typedef struct ListNode {
int data;
struct ListNode* next;
} ListNode;
```
## 函数声明
```c
ListNode* createNode(int data);
void insertNode(ListNode* head, ListNode* node, int index);
void deleteNode(ListNode* head, int index);
ListNode* searchNode(ListNode* head, int data);
void printList(ListNode* head);
```
## 函数实现
```c
#include <stdio.h>
#include <stdlib.h>
ListNode* createNode(int data) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
void insertNode(ListNode* head, ListNode* node, int index) {
ListNode* p = head;
for (int i = 0; i < index; i++) {
p = p->next;
}
node->next = p->next;
p->next = node;
}
void deleteNode(ListNode* head, int index) {
ListNode* p = head;
for (int i = 0; i < index; i++) {
p = p->next;
}
ListNode* target = p->next;
p->next = target->next;
free(target);
}
ListNode* searchNode(ListNode* head, int data) {
ListNode* p = head->next;
while (p != NULL) {
if (p->data == data) {
return p;
}
p = p->next;
}
return NULL;
}
void printList(ListNode* head) {
ListNode* p = head->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
```
通过实现上述函数,我们就可以快速创建、操作、遍历单链表,实现数据类型的封装与使用。
相关推荐
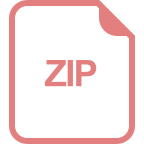
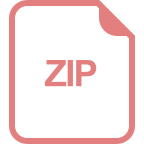
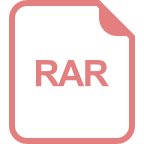














