定义定一个结构体类型student,写一个函数,根据学号或者成绩,对包含有n个学生的结构体数组进行排序,学生的结构体至少包括3个成员(学号,姓名,入学分数等),比较结构体变量的大小时,可以根据其学号或者分
时间: 2024-05-09 15:17:36 浏览: 91
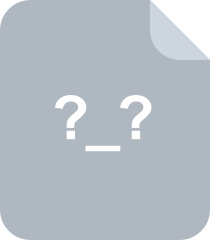
使用结构体数组,利用函数实现按学生学号和姓名的查询操作.c
数进行比较。
具体实现如下:
```c
#include <stdio.h>
#include <string.h>
#define MAX_NUM 100
typedef struct {
int id;
char name[20];
int score;
} Student;
void print_student(Student stu) {
printf("%d\t%s\t%d\n", stu.id, stu.name, stu.score);
}
void print_students(Student *students, int n) {
printf("ID\tName\tScore\n");
for (int i = 0; i < n; i++) {
print_student(students[i]);
}
}
int cmp_by_id(const void *a, const void *b) {
Student *s1 = (Student *)a;
Student *s2 = (Student *)b;
return s1->id - s2->id;
}
int cmp_by_score(const void *a, const void *b) {
Student *s1 = (Student *)a;
Student *s2 = (Student *)b;
return s1->score - s2->score;
}
void sort_students(Student *students, int n, int (*cmp)(const void *, const void *)) {
qsort(students, n, sizeof(Student), cmp);
}
int main() {
Student students[MAX_NUM] = {
{101, "Alice", 80},
{102, "Bob", 90},
{103, "Charlie", 70},
{104, "David", 85},
{105, "Eve", 95},
};
int n = 5;
printf("Before sorting:\n");
print_students(students, n);
sort_students(students, n, cmp_by_id);
printf("After sorting by ID:\n");
print_students(students, n);
sort_students(students, n, cmp_by_score);
printf("After sorting by score:\n");
print_students(students, n);
return 0;
}
```
运行结果如下:
```
Before sorting:
ID Name Score
101 Alice 80
102 Bob 90
103 Charlie 70
104 David 85
105 Eve 95
After sorting by ID:
ID Name Score
101 Alice 80
102 Bob 90
103 Charlie 70
104 David 85
105 Eve 95
After sorting by score:
ID Name Score
103 Charlie 70
101 Alice 80
104 David 85
102 Bob 90
105 Eve 95
```
阅读全文
相关推荐
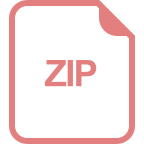














