python3 如何读写json
时间: 2023-09-28 09:07:46 浏览: 97
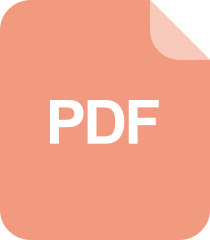
python读写json文件的简单实现
Python3 读写 JSON 的方法如下:
1.导入 json 库
可以通过导入 json 库来使用 json 格式:
```Python
import json
```
2.读取 JSON 文件
可以使用`json.load()`方法来读取 JSON 文件。首先,需要打开文件,然后使用`json.load()`方法将其加载到 Python 变量中。例如:
```Python
with open('data.json') as f:
data = json.load(f)
```
3.写入 JSON 文件
可以使用`json.dump()`方法来写入 JSON 文件。首先,需要将 Python 变量转换为 JSON 对象,然后使用`json.dump()`方法将其写入文件。例如:
```Python
data = {'name': 'Tom', 'age': 25, 'gender': 'male'}
with open('data.json', 'w') as f:
json.dump(data, f)
```
以上就是 Python3 读写 JSON 文件的方法。
阅读全文
相关推荐








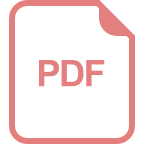
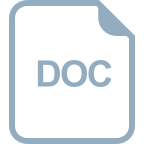
