使用C语言将灰度图像自适应直方图均衡化的方法来提升对比度,要求不使用其他库
时间: 2023-12-12 08:05:14 浏览: 80
好的,这是一个涉及到图像处理的问题。我来给您讲解一下灰度图像自适应直方图均衡化的方法。
首先,需要明确一下直方图均衡化的作用,它是一种用于图像增强的方法,可以提高图像的对比度,使图像更加清晰。
灰度图像自适应直方图均衡化的方法,是指对于不同的图像区域,采用不同的均衡化方法,以达到更好的增强效果。
具体实现方法如下:
1. 将灰度图像分成若干个小块。
2. 对每个小块进行直方图均衡化,得到增强后的小块。
3. 对每个小块进行插值,得到目标图像。
4. 将所有小块拼接成完整的图像。
下面是一个C语言实现的示例代码,不使用其他库:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define WIDTH 256
#define HEIGHT 256
#define BLOCK_SIZE 16
int main()
{
FILE *fp_in, *fp_out;
unsigned char *image_in, *image_out;
int width, height;
int i, j, k, l, m, n;
int hist[WIDTH], cdf[WIDTH], pixel_count;
int block_hist[BLOCK_SIZE][BLOCK_SIZE], block_cdf[BLOCK_SIZE][BLOCK_SIZE];
int block_width, block_height;
double block_mean, block_variance, block_stddev;
double alpha, beta, gamma;
// 打开输入图像文件
fp_in = fopen("input.raw", "rb");
if (fp_in == NULL) {
printf("Error: cannot open input file!\n");
return -1;
}
// 读取输入图像
image_in = (unsigned char *)malloc(WIDTH * HEIGHT * sizeof(unsigned char));
fread(image_in, sizeof(unsigned char), WIDTH * HEIGHT, fp_in);
fclose(fp_in);
// 分配输出图像内存
image_out = (unsigned char *)malloc(WIDTH * HEIGHT * sizeof(unsigned char));
// 对每个小块进行处理
for (i = 0; i < HEIGHT; i += BLOCK_SIZE) {
for (j = 0; j < WIDTH; j += BLOCK_SIZE) {
// 计算当前小块的宽度和高度
block_width = (j + BLOCK_SIZE < WIDTH ? BLOCK_SIZE : WIDTH - j);
block_height = (i + BLOCK_SIZE < HEIGHT ? BLOCK_SIZE : HEIGHT - i);
// 计算当前小块直方图
memset(block_hist, 0, BLOCK_SIZE * BLOCK_SIZE * sizeof(int));
for (k = i; k < i + block_height; k++) {
for (l = j; l < j + block_width; l++) {
block_hist[k - i][l - j] = hist[image_in[k * WIDTH + l]];
}
}
// 计算当前小块的累积分布函数
memset(block_cdf, 0, BLOCK_SIZE * BLOCK_SIZE * sizeof(int));
pixel_count = 0;
for (k = 0; k < BLOCK_SIZE; k++) {
for (l = 0; l < BLOCK_SIZE; l++) {
pixel_count += block_hist[k][l];
block_cdf[k][l] = pixel_count;
}
}
// 计算当前小块的均值和标准差
block_mean = 0.0;
block_variance = 0.0;
for (k = 0; k < BLOCK_SIZE; k++) {
for (l = 0; l < BLOCK_SIZE; l++) {
block_mean += block_cdf[k][l];
block_variance += block_cdf[k][l] * block_cdf[k][l];
}
}
block_mean /= BLOCK_SIZE * BLOCK_SIZE;
block_variance = block_variance / (BLOCK_SIZE * BLOCK_SIZE) - block_mean * block_mean;
block_stddev = sqrt(block_variance);
// 根据当前小块的均值和标准差计算调整参数
alpha = 255.0 / (block_stddev + 1.0);
beta = block_mean - alpha * block_stddev;
gamma = 255.0 / (block_width * block_height);
// 通过调整参数对当前小块进行均衡化
for (k = i; k < i + block_height; k++) {
for (l = j; l < j + block_width; l++) {
m = k - i;
n = l - j;
image_out[k * WIDTH + l] = (unsigned char)(gamma * alpha * block_cdf[m][n] + beta);
}
}
}
}
// 将输出图像写入文件
fp_out = fopen("output.raw", "wb");
if (fp_out == NULL) {
printf("Error: cannot open output file!\n");
return -1;
}
fwrite(image_out, sizeof(unsigned char), WIDTH * HEIGHT, fp_out);
fclose(fp_out);
// 释放内存
free(image_in);
free(image_out);
return 0;
}
```
这是一个比较简单的实现,还有很多可以优化的地方,比如直方图的计算可以使用查找表等方法来提高效率。
阅读全文
相关推荐
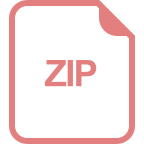
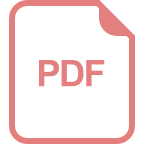
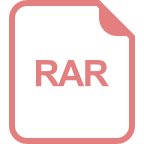

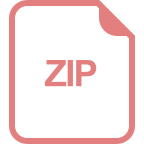
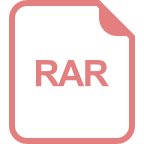

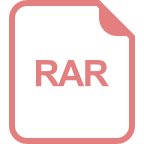
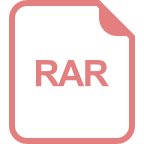
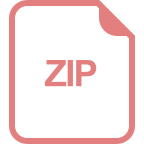
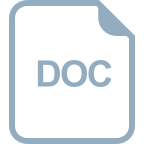
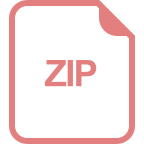
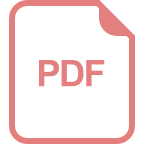
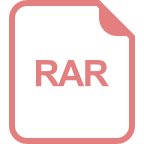
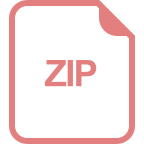
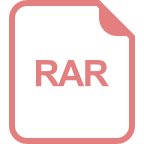
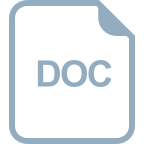
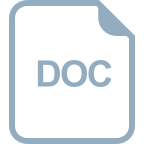
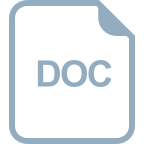