xlwings添加chart完整实例
时间: 2023-11-11 11:04:30 浏览: 122
下面是一个完整的xlwings添加chart的实例:
```python
import xlwings as xw
# 打开Excel文件并选择工作簿
wb = xw.Book('example.xlsx')
ws = wb.sheets['Sheet1']
# 添加一个新的图表
chart = ws.charts.add()
# 设置图表的类型
chart.chart_type = 'line'
# 设置图表的数据源
chart.set_source_data(ws.range('A1:B10'))
# 设置图表的标题和轴标签
chart.api[1].ChartTitle.Text = 'Chart Title'
chart.api[1].Axes(xlCategory).HasTitle = True
chart.api[1].Axes(xlCategory).AxisTitle.Text = 'X Axis Title'
chart.api[1].Axes(xlValue).HasTitle = True
chart.api[1].Axes(xlValue).AxisTitle.Text = 'Y Axis Title'
# 保存Excel文件
wb.save()
```
相关问题
xlwings添加chart完整示例代码
以下是使用xlwings创建图表的完整示例代码:
```python
import xlwings as xw
import numpy as np
# 创建一个新的Excel工作簿
wb = xw.Book()
# 在Sheet1中输入一些数据
sht = wb.sheets['Sheet1']
sht.range('A1').value = 'X'
sht.range('B1').value = 'Y'
sht.range('A2').value = np.arange(0, 10, 0.1)
sht.range('B2').value = np.sin(sht.range('A2').value)
# 创建一个图表对象并添加一个图表
chart = sht.charts.add()
chart.name = 'MyChart'
# 添加一个散点图系列
series = chart.series.add(sht.range('A2').expand(), sht.range('B2').expand())
series.name = 'Sin(x)'
series.marker.style = 'Circle'
# 添加一个线图系列
trendline = series.trendlines.add()
trendline.intercept.value = 0.5
trendline.display_equation = True
trendline.display_r_squared = True
# 设置图表标题和坐标轴标签
chart.api.HasTitle = True
chart.api.ChartTitle.Text = 'My Chart'
chart.api.Axes(xlCategory, xlPrimary).HasTitle = True
chart.api.Axes(xlCategory, xlPrimary).AxisTitle.Text = 'X'
chart.api.Axes(xlValue, xlPrimary).HasTitle = True
chart.api.Axes(xlValue, xlPrimary).AxisTitle.Text = 'Y'
# 调整图表大小和位置
chart.api.Parent.Top = 50
chart.api.Parent.Left = 50
chart.api.Parent.Width = 500
chart.api.Parent.Height = 300
# 保存Excel工作簿
wb.save('MyWorkbook.xlsx')
```
xlwings在chart中添加多条数据曲线实例
以下示例演示如何在Excel中添加多条数据曲线:
```
import xlwings as xw
import numpy as np
# 打开Excel文件
wb = xw.Book('example.xlsx')
# 选择工作表
sheet = wb.sheets['Sheet1']
# 生成x轴数据
x = np.linspace(0, 2*np.pi, 100)
# 添加第一条曲线
y1 = np.sin(x)
chart1 = sheet.charts.add()
chart1.set_source_data(sheet.range('A1:B100'))
chart1.chart_type = 'line'
chart1.set_x_axis({'name': 'X Axis'})
chart1.set_y_axis({'name': 'Y Axis'})
chart1.set_title({'name': 'Sin(x)'})
chart1.set_series(0, values=y1)
# 添加第二条曲线
y2 = np.cos(x)
chart2 = sheet.charts.add()
chart2.set_source_data(sheet.range('D1:E100'))
chart2.chart_type = 'line'
chart2.set_x_axis({'name': 'X Axis'})
chart2.set_y_axis({'name': 'Y Axis'})
chart2.set_title({'name': 'Cos(x)'})
chart2.set_series(0, values=y2)
# 关闭Excel文件
wb.close()
```
在上述示例中,我们首先打开Excel文件并选择要操作的工作表。然后,我们生成x轴数据,并使用`set_source_data`方法将数据范围设置为A1:B100和D1:E100。接着,我们添加两个图表对象并设置它们的类型、轴标签和标题。最后,我们使用`set_series`方法将每个曲线的数据添加到相应的图表中。
请注意,在`set_series`方法中,我们将`values`参数设置为`y1`和`y2`,这是我们在生成x轴数据后计算的两个曲线的数组。如果您有多个曲线,请将它们的数据添加到`values`参数中。
阅读全文
相关推荐
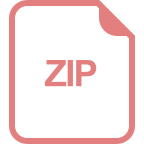










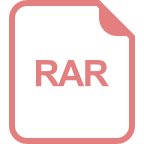
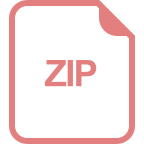

