用c++解决下述问题:描述 Akko is learning magic in Luna Nova Magical Academy. It is said that there are 10^5 types of different curses in the magic world. It is too hard for Akko to memorize all of them. Fortunately, Proffesor Meridies says that she can help Akko make a Curse Dictionary. When Akko hears a curse, the Dictionary will tell her the effect of the curse. Also, when Akko wants to do something, the Dictionary will find the curse for her. If there is no such curse, the Dictionary will be silence. Can you write a program to reproduce the Curse Dictionary? 输入 Each test case will contain no more than 10^5 curses. Each curse will be like "[name] effect". The name and effect of the curse will only contain lowercase letters. There will be only one space between ']' and the string after it. The length of the name will not exceed 20 and the length of the effect will not exceed 80. The end of the dictionary is a line of "END". After all curses there will be an integer N (1<=N<1000), followed by N queries. Every query will be a "[curse name]" or a "curse effect". There will be only one test case in each input file. 输出 For each test case, you must output the effect of the curse by its name or the name of the curse by its effect. If there is no curse satisfying the condition, print "silence". 提示 hashing strings gets() was deprecated in C++14, and use getline instead. for char arr[], use cin.getline(arr,sizeof(arr)); for string arr, use getline(cin, arr);
时间: 2024-02-14 10:10:41 浏览: 21
以下是一份可能的 C++ 代码实现:
```cpp
#include <iostream>
#include <unordered_map>
#include <string>
using namespace std;
int main() {
unordered_map<string, string> curses;
string curse;
while (getline(cin, curse)) {
if (curse == "END") {
break;
}
size_t pos = curse.find(']');
string name = curse.substr(1, pos - 1);
string effect = curse.substr(pos + 2);
curses[name] = effect;
}
int n;
cin >> n;
cin.ignore(); // 忽略上一个输入中的换行符
while (n--) {
string query;
getline(cin, query);
if (query[0] == '[') {
// 查询 curse name
string name = query.substr(1, query.size() - 2);
if (curses.count(name)) {
cout << curses[name] << endl;
} else {
cout << "silence" << endl;
}
} else {
// 查询 curse effect
bool found = false;
for (const auto& kvp : curses) {
if (kvp.second == query) {
cout << "[" << kvp.first << "]" << endl;
found = true;
break;
}
}
if (!found) {
cout << "silence" << endl;
}
}
}
return 0;
}
```
该程序首先使用 unordered_map 容器来存储所有的 curse name 和 curse effect 的对应关系。然后,根据输入的查询条件,遍历容器进行查询,并输出结果。如果查询条件不存在对应的 curse,输出 "silence"。
相关推荐
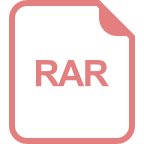
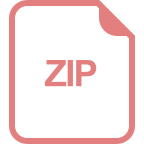


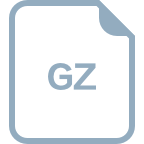
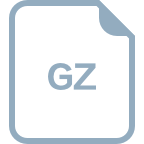
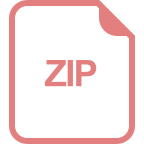
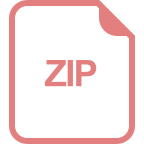
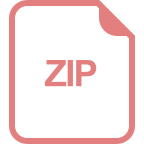
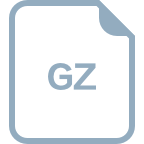
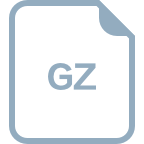
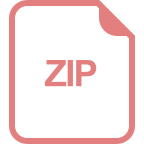