c语言实现替换文件中字符串
时间: 2024-03-20 17:39:47 浏览: 22
上面的回答已经给出了一个示例程序,演示了如何在 C 语言中实现替换文件中的字符串。
以下是一个稍微简化一些的示例程序,可以实现对文件中指定字符串的替换操作:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
const char* filename = "test.txt";
const char* old_str = "hello";
const char* new_str = "world";
FILE* fp = fopen(filename, "r");
if (fp == NULL) {
printf("Error: cannot open file %s\n", filename);
return EXIT_FAILURE;
}
fseek(fp, 0L, SEEK_END);
long file_size = ftell(fp);
rewind(fp);
char* file_content = malloc(file_size + 1);
if (file_content == NULL) {
printf("Error: cannot allocate memory for file content\n");
fclose(fp);
return EXIT_FAILURE;
}
size_t read_size = fread(file_content, 1, file_size, fp);
if (read_size != (size_t)file_size) {
printf("Error: cannot read file %s\n", filename);
free(file_content);
fclose(fp);
return EXIT_FAILURE;
}
fclose(fp);
file_content[file_size] = '\0';
char* old_pos = file_content;
while ((old_pos = strstr(old_pos, old_str)) != NULL) {
const size_t old_len = strlen(old_str);
const size_t new_len = strlen(new_str);
if (new_len > old_len) {
memmove(old_pos + new_len, old_pos + old_len, strlen(old_pos + old_len) + 1);
}
memcpy(old_pos, new_str, new_len);
old_pos += new_len;
}
fp = fopen(filename, "w");
if (fp == NULL) {
printf("Error: cannot open file %s for writing\n", filename);
free(file_content);
return EXIT_FAILURE;
}
fwrite(file_content, 1, file_size, fp);
fclose(fp);
free(file_content);
printf("Done!\n");
return EXIT_SUCCESS;
}
```
在这个示例程序中,我们首先打开要进行操作的文件,然后读取文件内容到内存中,使用 `strstr` 函数查找要替换的字符串,并使用 `memcpy` 函数执行替换操作。最后将修改后的文件内容写回到原文件中。
需要注意的是,为了避免内存泄漏,我们需要在程序中手动释放 `malloc` 函数分配的内存,以及关闭打开的文件句柄。
相关推荐
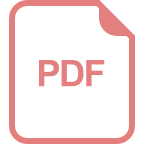














