用c++写一个指针链表,要求可以完成读取,删除,添加的功能
时间: 2024-05-29 20:12:12 浏览: 90
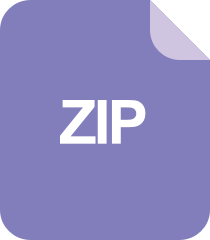
文件读写入链表.zip_C++链表_数据结构_文件读写_读链表_链表读取文本

#include <stdio.h>
#include <stdlib.h>
struct Node {
int data; // 存储数据
struct Node* next; // 指向下一个节点的指针
};
// 在链表末尾添加一个新节点
void addNode(struct Node** head, int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
// 如果链表为空,则将新节点设置为头节点
if (*head == NULL) {
*head = newNode;
return;
}
// 找到链表的末尾节点,并将其next指针指向新节点
struct Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
// 删除链表中第一个出现的指定节点
void deleteNode(struct Node** head, int data) {
// 如果链表为空,则直接返回
if (*head == NULL) {
return;
}
// 如果要删除的节点是头节点,则直接将头节点指向下一个节点
if ((*head)->data == data) {
struct Node* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
// 找到要删除的节点的前一个节点
struct Node* current = *head;
while (current->next != NULL && current->next->data != data) {
current = current->next;
}
// 如果找到了要删除的节点,则将其从链表中删除
if (current->next != NULL) {
struct Node* temp = current->next;
current->next = current->next->next;
free(temp);
}
}
// 输出链表中所有节点的值
void printList(struct Node* head) {
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
int main() {
struct Node* head = NULL;
// 添加节点
addNode(&head, 1);
addNode(&head, 2);
addNode(&head, 3);
addNode(&head, 4);
addNode(&head, 5);
// 输出链表
printf("Initial List: ");
printList(head);
// 删除节点
deleteNode(&head, 3);
deleteNode(&head, 1);
// 输出链表
printf("Updated List: ");
printList(head);
return 0;
}
阅读全文
相关推荐
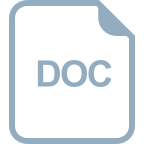
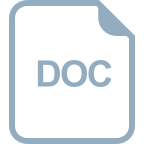
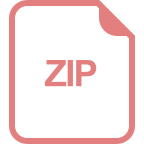
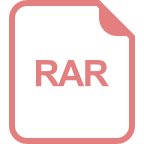
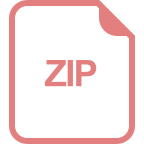
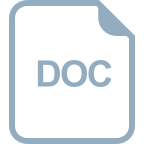










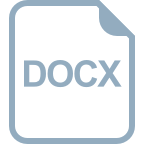
