c++用链表读取文件
时间: 2024-01-06 07:05:26 浏览: 251
在C++中,可以使用链表来读取文件。下面是一个示例代码,演示了如何使用链表读取文件中的数据:
```cpp
#include <iostream>
#include <fstream>
#include <string>
struct Node {
std::string data;
Node* next;
};
void readFileToList(Node*& head, const std::string& filename) {
std::ifstream file(filename);
if (file.is_open()) {
std::string line;
while (std::getline(file, line)) {
Node* newNode = new Node;
newNode->data = line;
newNode->next = nullptr;
if (head == nullptr) {
head = newNode;
} else {
Node* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
file.close();
} else {
std::cout << "Unable to open file: " << filename << std::endl;
}
}
void printList(const Node* head) {
const Node* current = head;
while (current != nullptr) {
std::cout << current->data << std::endl;
current = current->next;
}
}
int main() {
Node* head = nullptr;
std::string filename = "example.txt";
readFileToList(head, filename);
printList(head);
// 释放链表内存
Node* current = head;
while (current != nullptr) {
Node* temp = current;
current = current->next;
delete temp;
}
return 0;
}
```
这段代码首先定义了一个链表节点结构体`Node`,其中包含一个字符串数据成员`data`和一个指向下一个节点的指针`next`。
然后,`readFileToList`函数用于读取文件并将每一行数据存储到链表中。它首先打开文件,然后逐行读取文件内容。对于每一行,它创建一个新的节点,并将行数据存储到节点的`data`成员中。然后,它将新节点添加到链表的末尾。
最后,`printList`函数用于遍历链表并打印每个节点的数据。
在`main`函数中,我们首先定义了一个空链表头指针`head`,然后指定要读取的文件名`filename`。接下来,我们调用`readFileToList`函数将文件内容读取到链表中,并调用`printList`函数打印链表中的数据。
请注意,在使用完链表后,我们需要手动释放链表节点的内存,以避免内存泄漏。
阅读全文
相关推荐


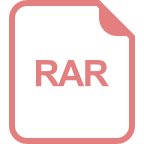











