c++语言,我已经完成了一个链表,头节点为head,如何将这个链表写入文件,并进行读取操作
时间: 2023-08-04 11:23:22 浏览: 42
要将链表写入文件,可以将链表节点中的数据依次写入文件中,可以用`ofstream`类来实现这一操作。具体步骤如下:
1. 打开文件,并将文件流对象与文件关联起来:
```
ofstream outfile("filename.txt");
```
2. 将链表节点中的数据写入文件中:
```
Node* p = head;
while (p != nullptr) {
outfile << p->data << endl;
p = p->next;
}
```
3. 关闭文件:
```
outfile.close();
```
要读取文件中的链表数据,可以按照以下步骤进行:
1. 打开文件,并将文件流对象与文件关联起来:
```
ifstream infile("filename.txt");
```
2. 读取文件中的数据,并将数据存储到链表节点中:
```
Node* p = head;
while (!infile.eof()) {
infile >> p->data;
p = p->next;
}
```
3. 关闭文件:
```
infile.close();
```
需要注意的是,在读取数据时,要判断文件是否已经读取到结尾(`eof()`函数),防止读取到文件末尾后出现错误。
相关问题
c++文件的读取和写入与链表
读取和写入文件可以使用C++中的文件流,具体可以使用`ifstream`和`ofstream`类。链表可以通过定义一个节点结构体,并在结构体中定义指向下一个节点的指针来实现。以下是一个读取文件并将内容存储在链表中的示例代码:
```c++
#include <iostream>
#include <fstream>
using namespace std;
struct Node {
int data;
Node* next;
};
int main() {
Node* head = nullptr;
Node* tail = nullptr;
ifstream inFile("data.txt");
int num;
while (inFile >> num) {
Node* newNode = new Node;
newNode->data = num;
newNode->next = nullptr;
if (head == nullptr) {
head = newNode;
tail = newNode;
} else {
tail->next = newNode;
tail = newNode;
}
}
inFile.close();
// 遍历链表
Node* cur = head;
while (cur != nullptr) {
cout << cur->data << " ";
cur = cur->next;
}
// 写入链表到文件
ofstream outFile("output.txt");
cur = head;
while (cur != nullptr) {
outFile << cur->data << " ";
cur = cur->next;
}
outFile.close();
// 释放链表内存
cur = head;
while (cur != nullptr) {
Node* temp = cur;
cur = cur->next;
delete temp;
}
return 0;
}
```
以上示例中,程序首先读取文件中的数据,并将数据存储在链表中。然后程序遍历链表并输出链表中的数据。最后程序将链表中的数据写入到文件中,并释放链表内存。
举例链表存入到文件怎么进行查找
好的,我来举一个简单的例子:
假设有如下链表:
```
struct Node {
int data;
Node* next;
};
Node* head = nullptr;
Node* tail = nullptr;
// 添加节点
void addNode(int data) {
Node* newNode = new Node();
newNode->data = data;
newNode->next = nullptr;
if (tail == nullptr) {
head = tail = newNode;
} else {
tail->next = newNode;
tail = newNode;
}
}
```
现在我们将这个链表存储到文件中,可以使用以下代码:
```
void saveListToFile(const std::string& filename) {
std::ofstream file(filename, std::ios::binary);
if (!file) {
std::cerr << "Failed to open file for writing: " << filename << std::endl;
return;
}
Node* node = head;
while (node != nullptr) {
file.write(reinterpret_cast<char*>(&node->data), sizeof(int));
node = node->next;
}
file.close();
}
```
这里使用 C++ 的文件流将链表数据写入文件。注意,我们使用了二进制模式打开文件,这样可以确保数据能够正确地写入和读取。
现在我们要从文件中查找某个数据,可以使用以下代码:
```
Node* findNode(int data) {
std::ifstream file("list.bin", std::ios::binary);
if (!file) {
std::cerr << "Failed to open file for reading: list.bin" << std::endl;
return nullptr;
}
Node* node = head;
int value;
while (file.read(reinterpret_cast<char*>(&value), sizeof(int))) {
if (value == data) {
return node;
}
node = node->next;
}
file.close();
return nullptr;
}
```
这里我们打开文件,从中读取数据,并遍历链表进行比较。如果找到了相等的节点,则返回该节点;否则返回 nullptr。
相关推荐







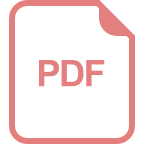
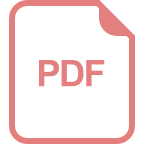
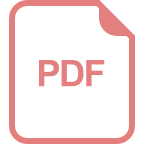
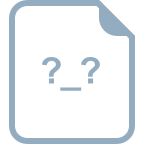