c++如何将链表写入一个文件中
时间: 2024-02-24 11:53:26 浏览: 186
在 C++ 中,可以使用 `fstream` 类来进行文件读写操作,其中 `ofstream` 类用于写入文件。
如果要将链表写入文件中,可以遍历链表并将每个节点的数据写入文件中。具体实现可以使用如下代码:
```c++
#include <fstream>
using namespace std;
struct Node {
int val;
Node* next;
Node(int x) : val(x), next(NULL) {}
};
void writeLinkedListToFile(Node* head, const string& filename) {
ofstream fout(filename);
if (fout.is_open()) {
Node* cur = head;
while (cur != NULL) {
fout << cur->val << " ";
cur = cur->next;
}
fout.close();
}
}
```
在上面的代码中,`writeLinkedListToFile` 函数将链表头节点指针 `head` 和要写入的文件名 `filename` 作为参数。函数遍历链表并将每个节点的值写入文件中,每个值之间用空格分隔。
假设我们有一个链表 `1 -> 2 -> 3 -> 4 -> NULL`,如果要将其写入文件 `example.txt` 中,可以使用如下代码:
```c++
Node* head = new Node(1);
head->next = new Node(2);
head->next->next = new Node(3);
head->next->next->next = new Node(4);
writeLinkedListToFile(head, "example.txt");
```
运行这段代码会将链表写入文件 `example.txt` 中,文件内容为:
```
1 2 3 4
```
阅读全文
相关推荐


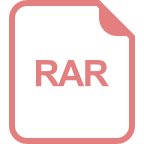














