写一份代码c++,将文件中的数据读取出来并存储到链表中,包括1打开文件,2 读取文件 3 关闭文件 和将链表中的数据存储到文件中1打开文件,2 读取文件 3 关闭文件
时间: 2024-02-29 18:53:17 浏览: 70
以下是c++代码实现:
```c++
#include <iostream>
#include <fstream>
using namespace std;
struct Node {
int data;
Node* next;
};
void readFile(Node*& head, const string& filename) {
ifstream file(filename);
if (file.is_open()) {
int value;
while (file >> value) {
Node* newNode = new Node;
newNode->data = value;
newNode->next = nullptr;
if (head == nullptr) {
head = newNode;
}
else {
Node* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
file.close();
cout << "File read successfully!" << endl;
}
else {
cout << "Error opening file!" << endl;
}
}
void writeFile(Node* head, const string& filename) {
ofstream file(filename);
if (file.is_open()) {
Node* current = head;
while (current != nullptr) {
file << current->data << endl;
current = current->next;
}
file.close();
cout << "File written successfully!" << endl;
}
else {
cout << "Error opening file!" << endl;
}
}
void printList(Node* head) {
Node* current = head;
while (current != nullptr) {
cout << current->data << " ";
current = current->next;
}
cout << endl;
}
int main() {
Node* head = nullptr;
const string filename = "data.txt";
// read file
readFile(head, filename);
// print list
cout << "Data in linked list: ";
printList(head);
// write file
writeFile(head, "output.txt");
// deallocate memory
Node* current = head;
while (current != nullptr) {
Node* temp = current;
current = current->next;
delete temp;
}
return 0;
}
```
这段代码实现了将文件中的数据读取出来并存储到链表中,以及将链表中的数据存储到文件中的功能。其中,`readFile`函数负责读取文件,`writeFile`函数负责写入文件,`printList`函数负责打印链表中的数据。需要注意的是,在读取完文件后,需要手动释放链表内存,避免内存泄漏。
阅读全文
相关推荐


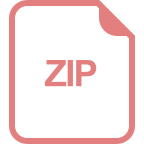
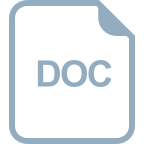






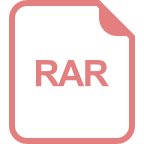
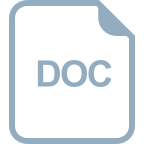



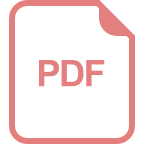
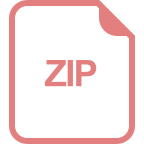