c++将文本文件转入到链表中
时间: 2024-06-11 20:07:19 浏览: 110
这里提供一个C语言的例子,可以将文本文件中的每一行读入到链表中:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct node {
char* data;
struct node* next;
} Node;
Node* create_node(char* data) {
Node* node = (Node*)malloc(sizeof(Node));
node->data = data;
node->next = NULL;
return node;
}
void free_list(Node* head) {
while (head != NULL) {
Node* temp = head;
head = head->next;
free(temp->data);
free(temp);
}
}
int main() {
FILE* fptr = fopen("data.txt", "r");
if (fptr == NULL) {
printf("Error: cannot open file.");
return 1;
}
Node* head = NULL;
Node* tail = NULL;
char buffer[100];
while (fgets(buffer, sizeof(buffer), fptr) != NULL) {
// remove newline character from the end of the line
buffer[strcspn(buffer, "\n")] = '\0';
char* data = (char*)malloc(strlen(buffer) + 1);
strcpy(data, buffer);
Node* node = create_node(data);
if (head == NULL) {
head = node;
} else {
tail->next = node;
}
tail = node;
}
fclose(fptr);
// print the list
Node* current = head;
while (current != NULL) {
printf("%s\n", current->data);
current = current->next;
}
// free the list
free_list(head);
return 0;
}
```
在此代码中,首先打开了一个名为`data.txt`的文件,然后逐行读取文件内容并将其插入到链表中。每个节点包含一个指向字符串数据的指针和一个指向下一个节点的指针。读取完成后,遍历链表并输出每个节点的字符串数据。最后,释放链表中的所有节点和字符串数据。
需要注意的是,在将字符串数据插入到链表中时,需要为每个字符串数据分配内存空间,并使用`strcpy()`函数将其复制到新分配的内存中。在释放链表时,需要先释放每个节点中的字符串数据,然后再释放节点本身。
阅读全文
相关推荐
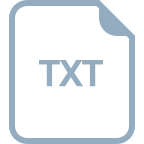
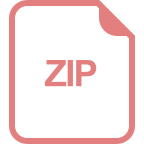
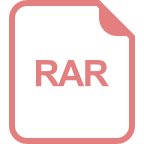
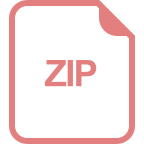
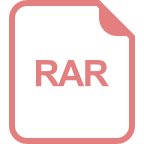
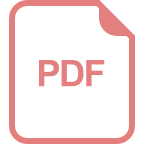
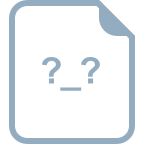
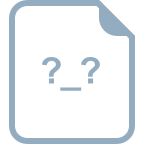
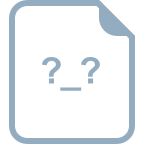
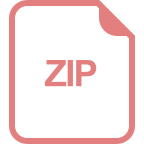
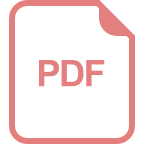
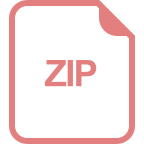
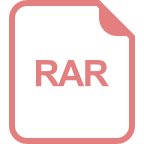
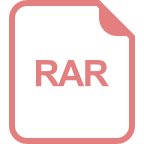
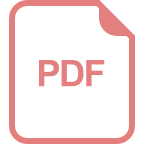
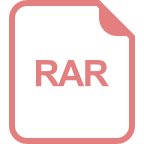