文件读取到链表
时间: 2023-07-18 21:11:56 浏览: 102
好的,您想了解如何将文件中的数据读取到链表中。一般来说,可以按照以下步骤进行:
1. 打开文件并读取数据:使用文件操作函数打开文件,然后逐行读取文件中的数据。
2. 将数据存储到链表中:对于每一行数据,可以创建一个节点,将数据存储到节点中,并将节点插入到链表中。
3. 关闭文件:在读取完文件中的所有数据后,关闭文件。
下面是一个C语言的示例代码,演示如何将文件中的数据读取到链表中:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义链表节点结构体
struct node {
char data[100];
struct node* next;
};
// 定义链表头指针
struct node* head = NULL;
// 读取文件数据到链表中
void read_file_to_list(char* file_name) {
FILE *fp = fopen(file_name, "r");
if(fp == NULL) {
printf("Failed to open file: %s\n", file_name);
return;
}
// 逐行读取文件中的数据
char line[100];
while(fgets(line, sizeof(line), fp)) {
// 创建新节点
struct node* new_node = (struct node*) malloc(sizeof(struct node));
strcpy(new_node->data, line);
new_node->next = NULL;
// 将新节点插入到链表中
if(head == NULL) {
head = new_node;
} else {
struct node* current = head;
while(current->next != NULL) {
current = current->next;
}
current->next = new_node;
}
}
fclose(fp);
}
int main() {
char* file_name = "data.txt";
read_file_to_list(file_name);
// 遍历链表输出数据
struct node* current = head;
while(current != NULL) {
printf("%s", current->data);
current = current->next;
}
return 0;
}
```
在上述代码中,我们使用 `fgets()` 函数逐行读取文件中的数据,然后将每行数据存储到链表节点中,最后遍历链表并输出数据。
阅读全文
相关推荐
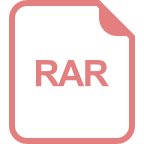



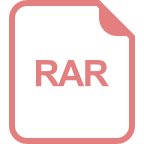
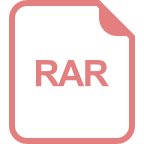








