c语言文件读取字符串到链表,请教C语言怎么把链表存入文件,从文件读取到链表...
时间: 2023-07-10 10:03:31 浏览: 129
将链表存入文件需要进行序列化操作,即将链表中的数据转化为二进制数据流,然后写入文件中。读取文件中的数据则需要进行反序列化操作,即将文件中的二进制数据流转化为链表中的数据。
下面是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct node {
char data[50];
struct node* next;
} Node;
void add(Node** head, char* data) {
Node* new_node = (Node*)malloc(sizeof(Node));
strcpy(new_node->data, data);
new_node->next = NULL;
if (*head == NULL) {
*head = new_node;
} else {
Node* cur = *head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = new_node;
}
}
void serialize(Node* head, char* filename) {
FILE* file = fopen(filename, "wb");
Node* cur = head;
while (cur != NULL) {
fwrite(cur->data, sizeof(char), strlen(cur->data) + 1, file);
cur = cur->next;
}
fclose(file);
}
Node* deserialize(char* filename) {
FILE* file = fopen(filename, "rb");
Node* head = NULL;
char data[50];
while (fread(data, sizeof(char), 50, file) != 0) {
add(&head, data);
}
fclose(file);
return head;
}
int main() {
Node* head = NULL;
add(&head, "hello");
add(&head, "world");
serialize(head, "data.bin");
Node* new_head = deserialize("data.bin");
Node* cur = new_head;
while (cur != NULL) {
printf("%s ", cur->data);
cur = cur->next;
}
printf("\n");
return 0;
}
```
代码中,`add`函数用于向链表中添加节点,`serialize`函数用于将链表序列化到文件中,`deserialize`函数用于从文件中反序列化出链表。
在序列化时,我们使用了`fwrite`函数将字符串数据写入文件中。
在反序列化时,我们使用了`fread`函数从文件中读取字符串数据,并调用`add`函数将其添加到链表中。
需要注意的是,在序列化和反序列化时,我们需要使用二进制文件模式(`"wb"`和`"rb"`),以确保在写入和读取数据时,不会将文本中的换行符等字符转换为其他字符,从而导致数据失真。
阅读全文
相关推荐
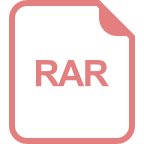
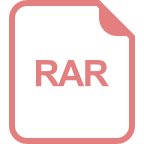
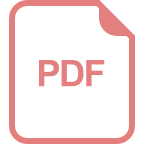
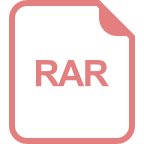
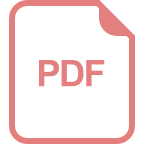
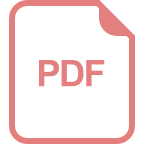
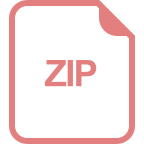
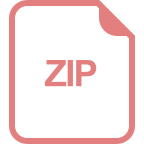
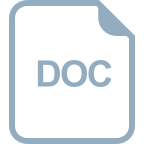
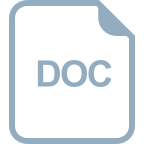
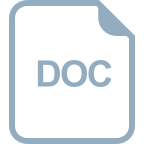
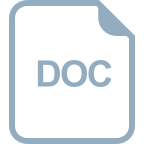
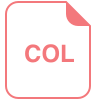




