c语言从一个文件读取到链表在写入另一个文件
时间: 2023-12-14 20:36:47 浏览: 82
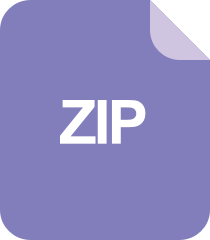
链表HuffmanTree-c语言文件读写
下面是一个示例代码,可以从一个文件中读取数据到链表中,然后将链表中的数据写入到另一个文件中:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表结构体
typedef struct Node {
int data;
struct Node *next;
} Node;
// 从文件中读取数据到链表中
Node* readFromFile(char* filename) {
FILE *fp = fopen(filename, "r");
if (fp == NULL) {
printf("Error opening file %s\n", filename);
return NULL;
}
Node *head = NULL;
Node *tail = NULL;
int data;
while (fscanf(fp, "%d", &data) != EOF) {
Node *node = (Node*)malloc(sizeof(Node));
node->data = data;
node->next = NULL;
if (head == NULL) {
head = tail = node;
} else {
tail->next = node;
tail = node;
}
}
fclose(fp);
return head;
}
// 将链表中的数据写入到文件中
void writeToFile(char* filename, Node *head) {
FILE *fp = fopen(filename, "w");
if (fp == NULL) {
printf("Error opening file %s\n", filename);
return;
}
Node *p = head;
while (p != NULL) {
fprintf(fp, "%d ", p->data);
p = p->next;
}
fclose(fp);
}
int main() {
Node *head = readFromFile("input.txt"); // 从 input.txt 文件中读取数据到链表中
writeToFile("output.txt", head); // 将链表中的数据写入到 output.txt 文件中
return 0;
}
```
在这个示例代码中,readFromFile() 函数从指定的文件中读取数据到链表中,writeToFile() 函数将链表中的数据写入到指定的文件中。在 main() 函数中,我们先调用 readFromFile() 函数将数据读取到链表中,然后再调用 writeToFile() 函数将链表中的数据写入到文件中。
需要注意的是,在使用完链表后,需要手动释放链表中的内存,以避免内存泄漏。可以使用如下的代码来释放链表中的内存:
```c
void freeList(Node *head) {
Node *p = head;
while (p != NULL) {
Node *tmp = p;
p = p->next;
free(tmp);
}
}
```
这个函数会遍历链表,释放每个节点的内存。在 main() 函数中,我们可以在程序结束前调用 freeList() 函数来释放链表中的内存:
```c
int main() {
Node *head = readFromFile("input.txt"); // 从 input.txt 文件中读取数据到链表中
writeToFile("output.txt", head); // 将链表中的数据写入到 output.txt 文件中
freeList(head); // 释放链表中的内存
return 0;
}
```
阅读全文
相关推荐
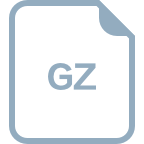
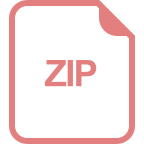















