OpenCV yolov5目标检测 返回X Y
时间: 2023-09-09 22:06:04 浏览: 54
使用OpenCV yolov5目标检测后,可以获取到检测到的目标的bounding box位置信息,其中包括左上角点的X坐标和Y坐标以及bounding box的宽度和高度。
具体来说,可以使用以下代码获取bounding box的信息:
```
import cv2
import numpy as np
# 加载模型
net = cv2.dnn.readNet("yolov5.weights", "yolov5.cfg")
# 加载类别标签
classes = []
with open("coco.names", "r") as f:
classes = [line.strip() for line in f.readlines()]
# 加载图像
img = cv2.imread("test.jpg")
# 获取输入图像的尺寸
height, width = img.shape[:2]
# 构建一个blob对象
blob = cv2.dnn.blobFromImage(img, 1/255.0, (416, 416), swapRB=True, crop=False)
# 将blob对象设置为输入
net.setInput(blob)
# 进行目标检测
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
outputs = net.forward(output_layers)
# 处理每个输出层
for output in outputs:
# 处理每个检测
for detection in output:
# 获取类别概率
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
# 过滤掉低置信度检测结果
if confidence > 0.5:
# 获取bounding box的位置信息
center_x = int(detection[0] * width)
center_y = int(detection[1] * height)
w = int(detection[2] * width)
h = int(detection[3] * height)
# 计算bounding box的左上角点的坐标
x = int(center_x - w / 2)
y = int(center_y - h / 2)
# 绘制bounding box
cv2.rectangle(img, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.putText(img, classes[class_id], (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示结果
cv2.imshow("Image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个示例代码中,我们使用OpenCV的dnn模块加载了一个yolov5的模型,并利用该模型完成了目标检测。我们首先将输入图像转换为blob对象,并将该对象设置为网络的输入。然后,我们从输出层获取了检测结果,并对每个检测进行处理,得到bounding box的位置信息。最后,我们将bounding box绘制在原始图像上,并显示结果。
相关推荐
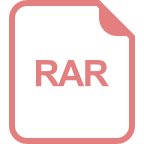
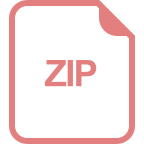
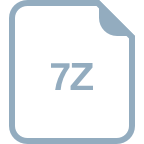














