导入自制成绩表,绘制柱形图(三组),x轴刻度标签为姓名,使用三种不同方法为三组柱形分别设置颜色(不要红色和绿色)。要有图例。 在此图上添加一组散点,大圆圈,散点y轴位置1,针对某一科目成绩做如下标记
时间: 2024-12-14 13:28:01 浏览: 2
在Python中,可以使用matplotlib库来完成这个任务。首先,假设你有一个包含学生姓名、三门课程分数的列表,你可以这样做:
```python
import matplotlib.pyplot as plt
import pandas as pd
# 假设你的成绩数据是这样的,存储在一个DataFrame中
data = {
'姓名': ['张三', '李四', '王五', '赵六', '孙七'],
'数学': [85, 90, 78, 88, 92],
'语文': [92, 88, 95, 86, 94],
'英语': [80, 85, 82, 87, 88]
}
df_scores = pd.DataFrame(data)
# 方法一:直接设置颜色(除红绿)
colors = ['blue', 'yellow', 'purple'] # 使用其他颜色代替红绿
fig, ax1 = plt.subplots()
# 绘制三组柱状图
bar1 = ax1.bar(df_scores['姓名'], df_scores['数学'], color=colors[0])
bar2 = ax1.bar(df_scores['姓名'], df_scores['语文'], color=colors[1], bottom=df_scores['数学'])
bar3 = ax1.bar(df_scores['姓名'], df_scores['英语'], color=colors[2], bottom=[df_scores.loc[i, '数学'] + df_scores.loc[i, '语文'] for i in range(len(df_scores))])
# 设置x轴标签和图例
ax1.set_xlabel('姓名')
ax1.set_ylabel('分数')
plt.xticks(rotation=45) # 设置x轴刻度标签倾斜
ax1.legend([bar1, bar2, bar3], ['数学', '语文', '英语'])
# 添加散点图
scatter_data = {'姓名': df_scores['姓名'].values, '特定科目': df_scores.iloc[:, -1].values}
scatter = ax1.scatter(scatter_data['姓名'], scatter_data['特定科目'], s=100, label='特殊标记', c='black') # 黑色散点
# 图例和标记特定科目
plt.legend(handles=[scatter], labels=['特定科目成绩'])
plt.axhline(y=1, color='gray', linestyle='dashed') # y轴刻度1的虚线
plt.text(0.5, 1.1, '某科目成绩', fontsize=12, transform=ax1.transAxes) # 显示标记信息
plt.show()
```
这里创建了一个柱状图,柱子的颜色分别为蓝、黄和紫,且设置了图例。然后添加了散点图,并对某一科目的成绩进行了特殊的标记。请注意,实际操作中你需要根据你的数据调整代码。
阅读全文
相关推荐
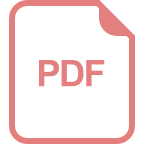
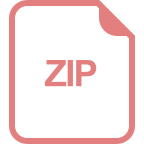
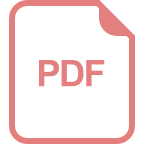





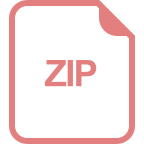
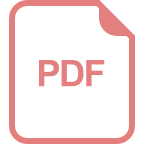
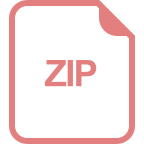
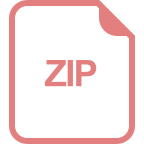
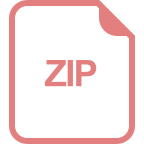
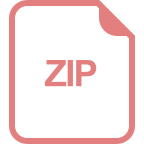
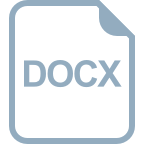
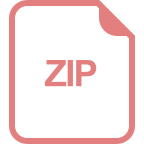
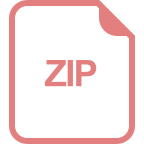