tf.keras.model
时间: 2023-11-09 07:45:47 浏览: 48
tf.keras.model是TensorFlow中的一个类,用于定义和训练神经网络模型。它提供了一种方便的方式来构建模型,包括定义输入和输出层、选择激活函数、设置优化器等。
通过引用[1]和,我们可以看到tf.keras.model的使用方法。首先,我们需要使用tf.keras.Input函数定义模型的输入层。然后,我们可以使用tf.keras.layers.Dense函数定义其他层,并将前一层的输出作为参数传递给当前层。最后,使用tf.keras.Model函数将输入和输出部分作为参数来创建模型。
另一种实例化tf.keras.model的方法是通过继承Model类来定义模型。我们可以定义模型的结构和层之间的连接关系,并实例化一个Model类对象。这种方法在引用中有详细介绍。
tf.keras.model还提供了一些属性,如metrics_names。这个属性返回模型的所有输出的显示标签。例如,我们可以使用model.metrics_names属性获取模型的损失和平均绝对误差的标签。引用中有相关示例代码。
总而言之,tf.keras.model是TensorFlow中用于定义和训练神经网络模型的类。我们可以使用它来构建模型、设置优化器和评估指标等。
相关问题
tf.keras.model.fit 多进程
在 TensorFlow 中,可以使用 `tf.keras.utils.multi_gpu_model` 函数将模型复制到多个 GPU 上进行并行训练。同时,也可以使用多进程来加速训练过程。
具体实现方式如下:
1. 导入必要的库和模块:
```python
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras import layers
import multiprocessing
```
2. 定义模型:
```python
def build_model():
inputs = keras.Input(shape=(784,))
x = layers.Dense(64, activation='relu')(inputs)
x = layers.Dense(64, activation='relu')(x)
outputs = layers.Dense(10, activation='softmax')(x)
model = keras.Model(inputs=inputs, outputs=outputs)
return model
```
3. 定义训练函数:
```python
def train(model, x_train, y_train, x_test, y_test, epochs):
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
model.fit(x_train, y_train, epochs=epochs, validation_data=(x_test, y_test))
```
4. 定义多进程训练函数:
```python
def train_multiprocess(model, x_train, y_train, x_test, y_test, epochs, num_processes):
strategy = tf.distribute.experimental.MultiWorkerMirroredStrategy()
with strategy.scope():
parallel_model = model
parallel_model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
train_dataset = tf.data.Dataset.from_tensor_slices((x_train, y_train)).batch(128)
test_dataset = tf.data.Dataset.from_tensor_slices((x_test, y_test)).batch(128)
options = tf.data.Options()
options.experimental_distribute.auto_shard_policy = tf.data.experimental.AutoShardPolicy.DATA
train_dataset = train_dataset.with_options(options)
test_dataset = test_dataset.with_options(options)
with multiprocessing.Pool(processes=num_processes) as pool:
for epoch in range(epochs):
train_results = pool.map(parallel_model.train_on_batch, train_dataset)
test_results = pool.map(parallel_model.test_on_batch, test_dataset)
train_loss = sum([result[0] for result in train_results]) / len(train_results)
train_acc = sum([result[1] for result in train_results]) / len(train_results)
test_loss = sum([result[0] for result in test_results]) / len(test_results)
test_acc = sum([result[1] for result in test_results]) / len(test_results)
print(f'Epoch {epoch+1}/{epochs}: train_loss={train_loss:.4f}, train_acc={train_acc:.4f}, test_loss={test_loss:.4f}, test_acc={test_acc:.4f}')
```
5. 加载数据和调用训练函数:
```python
(x_train, y_train), (x_test, y_test) = keras.datasets.mnist.load_data()
x_train = x_train.reshape((60000, 784)).astype('float32') / 255
x_test = x_test.reshape((10000, 784)).astype('float32') / 255
num_processes = 2 # 设置进程数
model = build_model()
train_multiprocess(model, x_train, y_train, x_test, y_test, epochs=10, num_processes=num_processes)
```
在训练过程中,每个进程将会使用一个单独的 GPU 来计算。如果希望使用多个 GPU,可以将 `tf.distribute.experimental.MultiWorkerMirroredStrategy` 替换为 `tf.distribute.MirroredStrategy`。如果希望使用更多进程,可以将 `num_processes` 参数增加。需要注意的是,增加进程数会增加 CPU 和内存的开销,可能会导致训练过程变慢。
tf.keras model.compile
tf.keras model.compile是TensorFlow深度学习框架中的一个函数,主要用于编译keras模型,定义模型的各种参数,以便进行训练和评估操作。
tf.keras model.compile函数有以下几个常用参数:
- optimizer:优化器,常见的有随机梯度下降法(SGD)、Adam、Adagrad等
- loss:损失函数,常见的有均方误差(MSE)、交叉熵(categorical_crossentropy)等
- metrics:度量指标,用于评估模型的性能,常见的有准确率(accuracy)、精确率(precision)、召回率(recall)等
- loss_weights:损失权重,用于为不同的损失函数赋予不同的权重
- sample_weight_mode:样本权重模式,通常是None或seqofdicts
- weighted_metrics:加权度量指标,为每个批次中的样本赋予不同的权重
通过调用tf.keras model.compile函数,我们可以定义模型的优化器、损失函数和评估指标等,并为模型提供各种参数,以便进行训练和评估操作。例:
```
model.compile(optimizer='adam',
loss='binary_crossentropy',
metrics=['accuracy'])
```
上述代码中,我们定义了一个使用Adam优化器、二元交叉熵损失函数和准确率度量指标的keras模型。这些参数将被用于训练和评估模型,以便根据数据集的不同要求计算模型的性能和准确度。
相关推荐
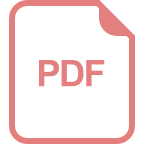
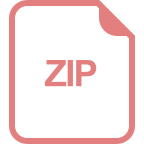
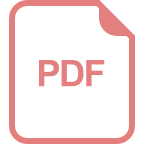












