词云图python完整代码
时间: 2024-04-19 19:22:55 浏览: 27
当使用Python生成词云图时,可以使用第三方库WordCloud来实现。以下是一个简单的示例代码:
```python
# 导入所需的库
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 读取文本文件
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 创建词云对象
wordcloud = WordCloud(width=800, height=400, background_color='white').generate(text)
# 绘制词云图
plt.figure(figsize=(10, 6))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
```
上述代码中,首先导入了WordCloud和matplotlib.pyplot库。然后使用`open()`函数读取文本文件,将其保存到`text`变量中。接下来,创建了一个词云对象`wordcloud`,并指定了词云图的宽度、高度和背景颜色。最后,使用`imshow()`函数绘制词云图,并使用`axis('off')`函数隐藏坐标轴,最后使用`show()`函数显示词云图。
相关问题
词云图python代码
以下是一个简单的词云图 Python 代码:
```python
import matplotlib.pyplot as plt
from wordcloud import WordCloud, STOPWORDS
# 读取文本文件
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 设置停用词
stopwords = set(STOPWORDS)
stopwords.add('said')
# 生成词云图
wordcloud = WordCloud(stopwords=stopwords, background_color='white', width=800, height=600).generate(text)
# 显示词云图
plt.figure(figsize=(12, 10))
plt.imshow(wordcloud)
plt.axis('off')
plt.show()
```
其中,`text.txt` 是要生成词云图的文本文件。`stopwords` 变量设置停用词,这些词不会出现在词云图中。`WordCloud` 类可以设置词云图的一些参数,例如停用词、背景颜色、宽度和高度等。最后,使用 `plt.imshow` 显示词云图。
产生词云图python代码
生成词云图(Word Cloud)是Python中常用的数据可视化方法之一,它通常用于展示文本数据中的高频词汇。使用Python库如`wordcloud`和`matplotlib`,你可以创建出美观的词云。以下是一个简单的步骤和代码示例:
首先,确保你已经安装了以下库:
```bash
pip install wordcloud matplotlib
```
然后,你可以用以下代码生成词云图:
```python
import matplotlib.pyplot as plt
from wordcloud import WordCloud
# 假设你有一个包含文本数据的字符串
text_data = """
这是一段包含文本的字符串,你可以根据实际需要替换为你的文本内容,例如文章摘要、社交媒体帖子、邮件等。
"""
# 设置词云参数
wordcloud = WordCloud(font_path='你的字体路径', # 如果需要特定字体
background_color='white',
width=800,
height=600,
max_words=200, # 显示的最大单词数量
stopwords=[]) # 需要去除的停用词列表
# 生成词云
wordcloud.generate(text_data)
# 显示词云图
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis("off")
plt.show()
```
在这里,你需要替换`font_path`为你计算机上可用的字体文件路径,并根据需要调整其他参数,如背景颜色、词云大小、显示的单词数量等。
相关推荐
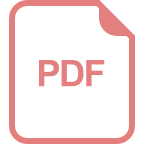
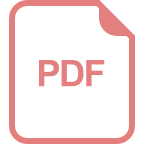

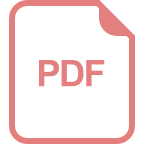
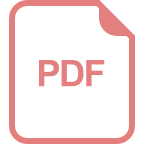
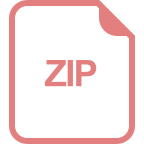
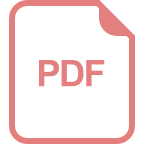
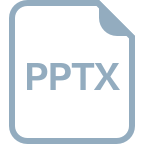
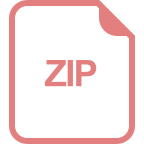
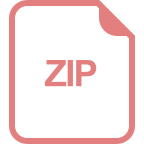
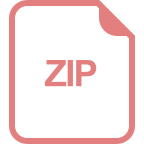
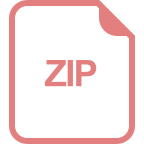