c++分割string
时间: 2023-11-24 10:47:04 浏览: 77
可以使用stringstream和getline函数来分割string,具体实现如下:
```c++
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using namespace std;
vector<string> split(const string& s, char delimiter) {
vector<string> tokens;
string token;
istringstream tokenStream(s);
while (getline(tokenStream, token, delimiter)) {
tokens.push_back(token);
}
return tokens;
}
int main() {
string s = "hello world";
vector<string> tokens = split(s, ' ');
for (const auto& token : tokens) {
cout << token << endl;
}
return 0;
}
```
输出结果为:
```
hello
world
```
相关问题
c++ 分割string
c分割string指的是在c编程语言中,对一个字符串进行分割操作,即将字符串按照特定的字符或字符串分割为多个子串。这个操作可以使用c语言中的字符串库中的函数来实现,如strtok()、strsep()、strstr()等。
其中,strtok()是最常用的字符串分割函数之一。它使用一个字符串作为分割符,将输入的字符串分割为多个子串,并返回第一个子串的指针。通过对返回指针进行不断迭代,即可逐个取得所有子串。strtok()函数每次调用时需要传入两个参数:1)需要被分割的字符串;2)用于分割的字符或字符串。
下面是一个使用strtok()函数分割字符串的示例代码:
```
#include <stdio.h>
#include <string.h>
int main()
{
char str[] = "apple,banana,orange,grape";
char *token;
token = strtok(str, ",");
while(token != NULL)
{
printf("%s\n", token);
token = strtok(NULL, ",");
}
return 0;
}
```
这段代码会将字符串str按照逗号分割为四个子串:apple、banana、orange、grape,并将每个子串逐个输出到控制台上。在实际应用中,字符串分割经常用于读取配置文件、解析文本数据等场景中。
C++分割string字符串
在 C++ 中,可以使用 `std::string` 类型和 `std::stringstream` 类型来分割字符串。
方法一:使用 `std::stringstream`
```cpp
#include <iostream>
#include <string>
#include <sstream>
#include <vector>
int main() {
std::string str = "hello world how are you";
std::stringstream ss(str);
std::vector<std::string> tokens;
std::string token;
while (std::getline(ss, token, ' ')) {
tokens.push_back(token);
}
for (const auto& t : tokens) {
std::cout << t << std::endl;
}
return 0;
}
```
输出:
```
hello
world
how
are
you
```
方法二:使用 `std::string` 的 `find` 函数和 `substr` 函数
```cpp
#include <iostream>
#include <string>
#include <vector>
int main() {
std::string str = "hello world how are you";
std::vector<std::string> tokens;
size_t pos = 0;
std::string token;
while ((pos = str.find(' ')) != std::string::npos) {
token = str.substr(0, pos);
tokens.push_back(token);
str.erase(0, pos + 1);
}
tokens.push_back(str);
for (const auto& t : tokens) {
std::cout << t << std::endl;
}
return 0;
}
```
输出:
```
hello
world
how
are
you
```
阅读全文
相关推荐
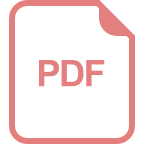













