c++string分割
时间: 2023-11-24 12:47:04 浏览: 33
可以使用stringstream和getline函数来实现C++中的字符串分割。具体实现方法如下:
```c++
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using namespace std;
vector<string> split(string str, char delimiter) {
vector<string> tokens;
stringstream ss(str);
string token;
while (getline(ss, token, delimiter)) {
tokens.push_back(token);
}
return tokens;
}
int main() {
string str = "hello world, this is a test";
vector<string> tokens = split(str, ' ');
for (auto token : tokens) {
cout << token << endl;
}
return 0;
}
```
输出结果为:
```
hello
world,
this
is
a
test
```
相关问题
c++ string分割
在C++中,有多种方法可以实现字符串分割。引用\[1\]中的代码使用了boost库的正则表达式来实现字符串分割。它定义了一个split函数,接受一个字符串和一个分隔符作为参数,然后使用boost库中的正则表达式来将字符串分割成多个子字符串,并将它们存储在一个vector中返回。
引用\[2\]中的代码使用了substr函数来实现字符串分割。它定义了一个split函数,接受一个字符串和一个分隔符作为参数,然后使用substr函数来截取子字符串,并将它们存储在一个vector中返回。
引用\[3\]中的代码使用了boost库的split函数来实现字符串分割。它定义了一个main函数,接受一个字符串作为输入,然后使用boost库中的split函数来将字符串按照指定的分隔符进行分割,并将分割后的子字符串存储在一个vector中返回。
所以,根据你的需求,你可以选择使用boost库的正则表达式或split函数,或者使用substr函数来实现字符串分割。具体选择哪种方法取决于你的具体需求和个人偏好。
#### 引用[.reference_title]
- *1* *2* *3* [C++如何做字符串分割(5种方法)](https://blog.csdn.net/birenxiaofeigg/article/details/115464934)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
c++ string 分割字符串
以下是两种C++中分割字符串的方法:
1. 使用stringstream和getline函数
```cpp
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
std::vector<std::string> splitString(std::string str, char delimiter) {
std::vector<std::string> result;
std::stringstream ss(str);
std::string token;
while (getline(ss, token, delimiter)) {
result.push_back(token);
}
return result;
}
int main() {
std::string str = "This is a test";
std::vector<std::string> strList = splitString(str, ' ');
for (auto s : strList) {
std::cout << s << " ";
}
std::cout << std::endl;
return 0;
}
```
2. 使用find和substr方法
```cpp
#include <iostream>
#include <string>
#include <vector>
std::vector<std::string> splitString(std::string str, char delimiter) {
std::vector<std::string> result;
size_t start = 0;
size_t end = str.find(delimiter);
while (end != std::string::npos) {
result.push_back(str.substr(start, end - start));
start = end + 1;
end = str.find(delimiter, start);
}
result.push_back(str.substr(start, end));
return result;
}
int main() {
std::string str = "This-is-a-test";
std::vector<std::string> strList = splitString(str, '-');
for (auto s : strList) {
std::cout << s << " ";
}
std::cout << std::endl;
return 0;
}
```
相关推荐
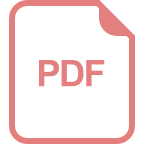
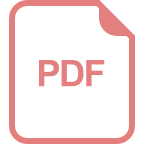
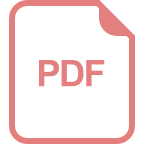












