c++ string空格分割字符串
时间: 2023-04-11 13:00:51 浏览: 207
可以使用 stringstream 和 getline 函数来实现空格分割字符串,示例代码如下:
```c++
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using namespace std;
int main() {
string s = "hello world";
stringstream ss(s);
vector<string> tokens;
string token;
while (getline(ss, token, ' ')) {
tokens.push_back(token);
}
for (const auto& t : tokens) {
cout << t << endl;
}
return 0;
}
```
输出结果为:
```
hello
world
```
相关问题
c++按空格分割字符串
要按空格分割字符串,可以使用以下几种方法:
1. 使用istringstream和getline()函数:
```
#include <iostream>
#include <sstream>
#include <vector>
int main() {
std::string str = "abc def ghi";
std::istringstream ss(str);
std::string token;
std::vector<std::string> tokens;
while(std::getline(ss, token, ' ')) {
tokens.push_back(token);
}
for(const auto& token : tokens) {
std::cout << token << std::endl;
}
return 0;
}
```
这段代码使用istringstream和getline()函数来逐个读取字符串中的单词,并将它们存储在一个vector中。
2. 使用C风格字符串中的strtok()函数:
```
#include <iostream>
#include <cstring>
#include <vector>
int main() {
std::string str = "abc def ghi";
char* dup = strdup(str.c_str());
char* token = strtok(dup, " ");
std::vector<std::string> tokens;
while(token != NULL) {
tokens.push_back(std::string(token));
token = strtok(NULL, " ");
}
free(dup);
for(const auto& token : tokens) {
std::cout << token << std::endl;
}
return 0;
}
```
这段代码使用strtok()函数将字符串分割成单词,并将它们存储在一个vector中。需要注意的是,由于strtok()函数修改了原始字符串,我们需要首先创建一个副本,然后在使用完毕后释放它。
3. 使用find和substr函数:
```
#include <iostream>
#include <string>
#include <vector>
int main() {
std::string str = "abc def ghi";
std::vector<std::string> tokens;
std::size_t pos = 0;
std::string delimiter = " ";
while((pos = str.find(delimiter)) != std::string::npos) {
std::string token = str.substr(0, pos);
tokens.push_back(token);
str.erase(0, pos + delimiter.length());
}
tokens.push_back(str);
for(const auto& token : tokens) {
std::cout << token << std::endl;
}
return 0;
}
```
这段代码使用find和substr函数来逐个查找并提取字符串中的单词,并将它们存储在一个vector中。通过不断更新pos和擦除已提取的部分,我们可以实现字符串的分割。
以上是三种常用的在C++中按空格分割字符串的方法。根据实际需求和个人喜好,可以选择其中任意一种方法来实现。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [C++如何进行字符串分割,C++如何按照空格对字符串进行解析,C++如何按照逗号对字符串解析](https://blog.csdn.net/Sansipi/article/details/127597697)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
c++ 字符串空格分割字符串
可以使用 stringstream 和 getline 函数来实现字符串的空格分割。具体实现如下:
```c++
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using namespace std;
int main() {
string s = "hello world, how are you?";
stringstream ss(s);
string token;
vector<string> tokens;
while (getline(ss, token, ' ')) {
tokens.push_back(token);
}
for (auto t : tokens) {
cout << t << endl;
}
return 0;
}
```
上述代码中,我们首先定义了一个字符串 s,然后使用 stringstream 将其转换为一个流对象 ss。接着,我们定义了一个字符串变量 token 和一个字符串向量 tokens,然后使用 getline 函数从 ss 中逐个读取以空格为分隔符的字符串,并将其存储到 tokens 中。最后,我们遍历 tokens 并输出其中的每个字符串。
相关推荐
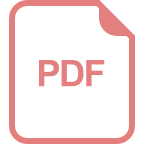
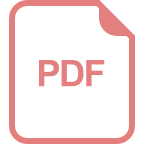
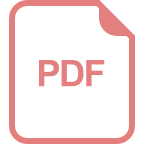












