C++根据字符分割字符串
时间: 2024-02-24 22:26:00 浏览: 26
你可以使用C语言中的字符串处理函数(string.h库)中的strtok函数来实现根据字符分割字符串。例如,如果你想根据空格分割字符串,可以使用以下代码:
```
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello World! It's a beautiful day.";
char* token = strtok(str, " ");
while (token != NULL) {
printf("%s\n", token);
token = strtok(NULL, " ");
}
return 0;
}
```
这个程序会把字符串str按照空格分割成若干个子串,并逐个输出它们。strtok函数的第一个参数为要分割的字符串,第二个参数为分割符号。在第一次调用strtok时,它会返回分割出的第一个子串,并把分割符号后面的部分替换成'\0',以方便后续处理。当再次调用strtok时,它会在上一次分割的位置继续分割,直到分割完整个字符串。
相关问题
c++字符串根据字符分割
可以使用C++的STL库中的stringstream和getline函数来实现字符串根据字符分割的功能。具体实现如下:
```c++
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
int main() {
std::string str = "This is a sample string.";
std::vector<std::string> tokens;
// 使用stringstream分割字符串
std::stringstream ss(str);
std::string token;
char delimiter = ' '; // 分割符为空格
while (std::getline(ss, token, delimiter)) {
tokens.push_back(token);
}
// 输出分割后的结果
for (const auto& t : tokens) {
std::cout << t << std::endl;
}
return 0;
}
```
代码中首先定义了一个字符串和一个字符串向量,然后使用stringstream将字符串按照指定的分割符进行分割,并将分割后的子字符串存储到向量中。最后遍历向量输出分割后的结果。
C++ 根据符号分割字符串
可以使用 C++ 标准库中的 `stringstream` 和 `getline` 函数来实现根据符号分割字符串的操作。具体实现如下:
```c++
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using namespace std;
int main() {
string str = "a,b,c,d,e";
char delim = ',';
vector<string> tokens;
stringstream ss(str);
string token;
while (getline(ss, token, delim)) {
tokens.push_back(token);
}
for (const auto& t : tokens) {
cout << t << endl;
}
return 0;
}
```
在上述代码中,我们将需要分割的字符串 `str` 和分隔符 `delim`(在本例中为逗号)定义好,并创建了一个空的字符串向量 `tokens`。接下来,我们使用 `stringstream` 将字符串 `str` 转化为一个流对象 `ss`。然后,我们使用 `getline` 函数从流中逐个读取分隔符之间的字符串,并将其添加到向量 `tokens` 中。
最后,我们使用一个 for 循环遍历向量 `tokens` 并将其中的每个字符串打印出来。
相关推荐
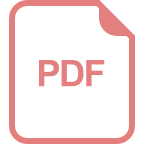












