springboot-jdbc查clickhouse
时间: 2023-11-27 08:47:25 浏览: 151
以下是使用Spring Boot结合MyBatis和Alibaba Druid连接池对ClickHouse进行数据操作的步骤:
1.在pom.xml文件中添加ClickHouse JDBC驱动和Alibaba Druid连接池的依赖:
```xml
<dependency>
<groupId>ru.yandex.clickhouse</groupId>
<artifactId>clickhouse-jdbc</artifactId>
<version>0.3.1</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.1.10</version>
</dependency>
```
2.在application.properties文件中配置ClickHouse和Druid的连接信息:
```properties
# ClickHouse 数据库连接配置
spring.datasource.url=jdbc:clickhouse://localhost:8123/default
spring.datasource.driver-class-name=ru.yandex.clickhouse.ClickHouseDriver
spring.datasource.username=username
spring.datasource.password=password
# Druid 连接池配置
spring.datasource.type=com.alibaba.druid.pool.DruidDataSource
spring.datasource.initial-size=1
spring.datasource.min-idle=1
spring.datasource.max-active=20
spring.datasource.max-wait=60000
spring.datasource.time-between-eviction-runs-millis=60000
spring.datasource.min-evictable-idle-time-millis=300000
spring.datasource.validation-query=SELECT 1 FROM DUAL
spring.datasource.test-while-idle=true
spring.datasource.test-on-borrow=false
spring.datasource.test-on-return=false
spring.datasource.pool-prepared-statements=true
spring.datasource.max-pool-prepared-statement-per-connection-size=20
spring.datasource.filters=stat,wall,log4j
spring.datasource.connection-properties=druid.stat.mergeSql=true;druid.stat.slowSqlMillis=5000
```
3.在Spring Boot的启动类上添加注解@EnableTransactionManagement和@MapperScan:
```java
@SpringBootApplication
@EnableTransactionManagement
@MapperScan("com.example.demo.mapper")
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
```
4.创建Mapper接口和对应的XML文件,使用MyBatis进行数据操作:
```java
@Mapper
public interface UserMapper {
@Select("SELECT * FROM user")
List<User> selectAll();
}
```
```xml
<mapper namespace="com.example.demo.mapper.UserMapper">
<resultMap id="BaseResultMap" type="com.example.demo.entity.User">
<id column="id" property="id" jdbcType="INTEGER"/>
<result column="name" property="name" jdbcType="VARCHAR"/>
<result column="age" property="age" jdbcType="INTEGER"/>
</resultMap>
<select id="selectAll" resultMap="BaseResultMap">
SELECT * FROM user
</select>
</mapper>
```
5.在Controller中注入Mapper并进行数据操作:
```java
@RestController
public class UserController {
@Autowired
private UserMapper userMapper;
@GetMapping("/users")
public List<User> getUsers() {
return userMapper.selectAll();
}
}
```
阅读全文
相关推荐
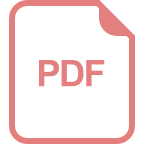
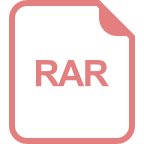





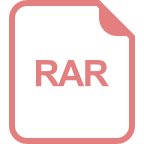
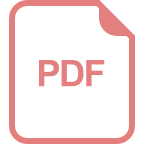








