React如何使用echarts将数据显示在百度地图上,代码实现
时间: 2024-05-16 22:12:23 浏览: 20
首先,需要安装 echarts 和百度地图组件 。
然后可以按照以下步骤进行操作:
1. 引入百度地图组件和 echarts
```javascript
import React, { Component } from 'react';
import { Map, PointCollection } from 'react-bmap';
import echarts from 'echarts/lib/echarts';
import 'echarts/lib/chart/bar';
import 'echarts/lib/component/tooltip';
import 'echarts/lib/component/title';
import 'echarts/map/js/china';
```
2. 在 componentDidMount 周期中初始化百度地图和 echarts
```javascript
componentDidMount() {
const { BMap } = window;
// 初始化百度地图
this.map = new BMap.Map('allmap');
this.map.centerAndZoom(new BMap.Point(116.404, 39.915), 11);
// 初始化echarts
echarts.registerMap('china', window.chinaJson);
this.chart = echarts.init(this.bmap.getMap());
// 设置地图的自定义样式
const styleJson = [
{
featureType: 'water',
elementType: 'all',
stylers: {
color: '#d1d1d1',
},
},
{
featureType: 'land',
elementType: 'all',
stylers: {
color: '#f3f3f3',
},
},
{
featureType: 'railway',
elementType: 'all',
stylers: {
visibility: 'off',
},
},
{
featureType: 'highway',
elementType: 'all',
stylers: {
color: '#fdfdfd',
},
},
{
featureType: 'highway',
elementType: 'labels',
stylers: {
visibility: 'off',
},
},
{
featureType: 'arterial',
elementType: 'geometry',
stylers: {
color: '#fefefe',
},
},
{
featureType: 'arterial',
elementType: 'geometry.fill',
stylers: {
color: '#fefefe',
},
},
{
featureType: 'poi',
elementType: 'all',
stylers: {
visibility: 'off',
},
},
{
featureType: 'green',
elementType: 'all',
stylers: {
visibility: 'off',
},
},
{
featureType: 'subway',
elementType: 'all',
stylers: {
visibility: 'off',
},
},
{
featureType: 'manmade',
elementType: 'all',
stylers: {
color: '#d1d1d1',
},
},
{
featureType: 'local',
elementType: 'all',
stylers: {
color: '#d1d1d1',
},
},
{
featureType: 'arterial',
elementType: 'labels',
stylers: {
visibility: 'off',
},
},
{
featureType: 'boundary',
elementType: 'all',
stylers: {
color: '#fefefe',
},
},
{
featureType: 'building',
elementType: 'all',
stylers: {
color: '#d1d1d1',
},
},
{
featureType: 'label',
elementType: 'labels.text.fill',
stylers: {
visibility: 'off',
},
},
];
this.map.setMapStyle({styleJson});
//加载地图后渲染图表
this.chart.setOption({
series: [
{
type: 'bar',
data: [5, 20, 36, 10, 10, 20]
}
]
});
}
```
3. 将 echarts 图表绑定到百度地图上
```javascript
class MyMap extends Component {
render() {
return (
<div id="allmap" style={{height: '400px'}}>
<Map
ref={(ref) => {
this.bmap = ref && ref.instance;
if (this.bmap && this.chart) {
this.chart.setOption({
xAxis: {
type: 'category',
data: ['Shanghai', 'Beijing', 'Guangzhou', 'Shenzhen', 'Hangzhou', 'Wuhan']
},
yAxis: {
type: 'value'
},
series: [
{
type: 'bar',
data: [5, 20, 36, 10, 10, 20],
barCategoryGap: '50%',
itemStyle: {
normal: {color: '#ddb926'}
}
}
]
});
this.chart.resize();
}
}}
>
{this.chart && (
<PointCollection
data={[...]}
options={{
...(this.props.pointOptions || {})
}}
onClick={(e) => {
const { target: point } = e, { dataIndex } = point;
console.log('[CLICK]', 'Index:', dataIndex);
// Set current index
this.setState(() => ({ currentIndex: dataIndex }));
// Highlight current points
this.pointCollection.trigger('highlight', { dataIndex });
this.pointCollection.trigger('moveTo', {
dataIndex,
duration: 500,
zoom: 16
});
}}
onMouseover={({ target: point }) => {
const { dataIndex } = point;
console.log('[HIGHLIGHT]', 'Index:', dataIndex);
this.pointCollection.trigger('highlight', { dataIndex });
}}
onMouseout={() => {
console.log('CANCEL HIGHLIGHT');
this.pointCollection.trigger('cancelHighlight');
}}
ref={(ref) => {
this.pointCollection = ref;
}}
/>
)}
</Map>
</div>
);
}
}
export default MyMap;
```
在以上代码中,我们创建了一个 MyMap 组件,通过在 Map 组件上渲染 PointCollection,可以将地图上的经纬度信息传递给 echarts 图表实例。最后,通过监听事件(如点击、鼠标移入等)来实现表格和地图之间的联动效果。
相关推荐
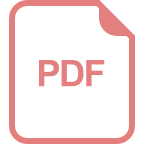
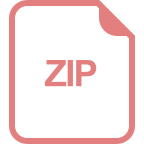
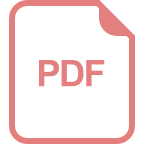














