mfc计算器b表达式求值
时间: 2023-07-08 12:43:32 浏览: 95
MFC计算器可以通过使用栈来实现表达式的求值。具体步骤如下:
1. 将中缀表达式转换为后缀表达式。
2. 创建一个栈,用来存储数字和运算符。
3. 从左到右扫描后缀表达式中的每个元素。
4. 如果元素是数字,则将其压入栈中。
5. 如果元素是运算符,则从栈中弹出两个数字进行运算,并将运算结果压入栈中。
6. 重复步骤 3 - 5,直到所有元素都被处理完毕。
7. 最后栈中只剩下一个元素,即为表达式的计算结果。
下面是一个简单的 MFC 计算器的代码,实现了上述步骤:
```
#include "stdafx.h"
#include "ExpressionEvaluator.h"
#include <stack>
double ExpressionEvaluator::Evaluate(const CString& expression)
{
CString postfix = InfixToPostfix(expression);
std::stack<double> stack;
double result = 0.0;
for (int i = 0; i < postfix.GetLength(); i++)
{
char c = postfix[i];
if (isdigit(c))
{
stack.push(c - '0');
}
else if (c == '+' || c == '-' || c == '*' || c == '/')
{
double a = stack.top();
stack.pop();
double b = stack.top();
stack.pop();
switch (c)
{
case '+':
result = b + a;
break;
case '-':
result = b - a;
break;
case '*':
result = b * a;
break;
case '/':
result = b / a;
break;
}
stack.push(result);
}
}
result = stack.top();
stack.pop();
return result;
}
CString ExpressionEvaluator::InfixToPostfix(const CString& expression)
{
CString postfix;
std::stack<char> stack;
for (int i = 0; i < expression.GetLength(); i++)
{
char c = expression[i];
if (isdigit(c))
{
postfix += c;
}
else if (c == '(')
{
stack.push(c);
}
else if (c == ')')
{
while (!stack.empty() && stack.top() != '(')
{
postfix += stack.top();
stack.pop();
}
if (!stack.empty() && stack.top() == '(')
{
stack.pop();
}
}
else if (c == '+' || c == '-' || c == '*' || c == '/')
{
while (!stack.empty() && GetPrecedence(c) <= GetPrecedence(stack.top()))
{
postfix += stack.top();
stack.pop();
}
stack.push(c);
}
}
while (!stack.empty())
{
postfix += stack.top();
stack.pop();
}
return postfix;
}
int ExpressionEvaluator::GetPrecedence(char c)
{
if (c == '+' || c == '-')
{
return 1;
}
else if (c == '*' || c == '/')
{
return 2;
}
else
{
return 0;
}
}
```
其中,`Evaluate` 函数用于计算表达式的值,`InfixToPostfix` 函数用于将中缀表达式转换为后缀表达式,`GetPrecedence` 函数用于获取运算符的优先级。
阅读全文
相关推荐
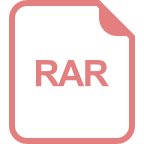
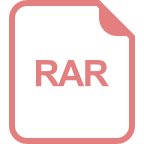
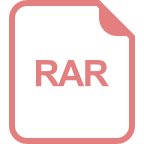
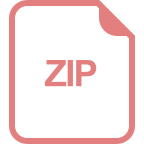
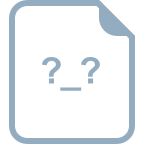
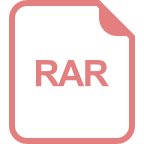
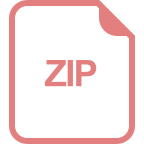
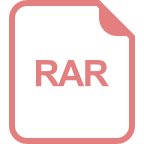
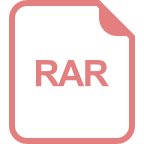
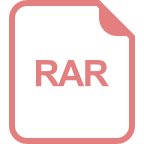
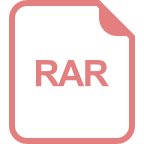
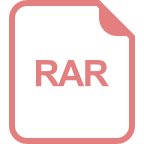
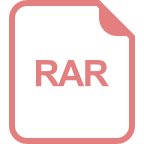