序贯航迹关联算法Matlab代码
时间: 2024-01-03 22:26:05 浏览: 150
以下是一个简单的基于Matlab的序贯航迹关联算法的实现示例:
```matlab
function [assignments, unassignedTracks, unassignedDetections] = ...
detectionToTrackAssignment(costMatrix, costOfNonAssignment)
% 初始化输入参数
[numTracks, numDetections] = size(costMatrix);
assignments = zeros(1, numTracks);
unassignedTracks = [];
unassignedDetections = [];
% 对于每个跟踪器,选择最小代价的检测,并将其分配给该跟踪器
[cost, trackIndices] = min(costMatrix, [], 2);
for i = 1:numTracks
% 如果最小代价大于阈值,则认为该跟踪器未匹配到检测
if cost(i) > costOfNonAssignment
unassignedTracks = [unassignedTracks i];
else
assignments(i) = trackIndices(i);
end
end
% 对于未分配的检测,选择最小代价的跟踪器,并将其分配给该检测
unassignedDetections = find(assignments==0);
for i = unassignedDetections
[~, trackIndex] = min(costMatrix(:,i));
if cost(trackIndex) <= costOfNonAssignment
assignments(trackIndex) = i;
else
unassignedTracks = [unassignedTracks trackIndex];
end
end
end
function tracks = updateAssignedTracks(assignments,centroids,bboxes,scores,tracks)
numAssignedTracks = size(assignments, 2);
for i = 1:numAssignedTracks
trackIdx = assignments(1, i);
detectionIdx = assignments(2, i);
centroid = centroids(detectionIdx, :);
bbox = bboxes(detectionIdx, :);
score = scores(detectionIdx);
% 更新跟踪器状态
tracks(trackIdx).centroid = centroid;
tracks(trackIdx).bbox = bbox;
tracks(trackIdx).score = score;
tracks(trackIdx).age = tracks(trackIdx).age + 1;
tracks(trackIdx).consecutiveInvisibleCount = 0;
end
end
function tracks = updateUnassignedTracks(unassignedTracks,tracks)
for i = 1:length(unassignedTracks)
ind = unassignedTracks(i);
tracks(ind).age = tracks(ind).age + 1;
tracks(ind).consecutiveInvisibleCount = ...
tracks(ind).consecutiveInvisibleCount + 1;
end
end
function [tracks,nextId] = createNewTracks(unassignedDetections,centroids,bboxes,scores,tracks,nextId)
centroids = centroids(unassignedDetections, :);
bboxes = bboxes(unassignedDetections, :);
scores = scores(unassignedDetections);
for i = 1:size(centroids, 1)
centroid = centroids(i,:);
bbox = bboxes(i, :);
score = scores(i);
% 创建一个新跟踪器
newTrack = struct(...
'id', nextId, ...
'centroid', centroid, ...
'bbox', bbox, ...
'score', score, ...
'age', 1, ...
'consecutiveInvisibleCount', 0);
% 将新跟踪器添加到跟踪器列表
tracks(end + 1) = newTrack;
% 增加下一个可用ID
nextId = nextId + 1;
end
end
function [tracks, nextId] = deleteLostTracks(tracks, nextId)
if isempty(tracks)
return;
end
invisibleForTooLong = 20;
ageThreshold = 8;
ages = [tracks(:).age]';
totalVisibleCounts = [tracks(:).totalVisibleCount]';
visibility = totalVisibleCounts ./ ages;
% 删除连续不可见时间超过阈值或者可见次数超过阈值但是跟踪时间太短的跟踪器
lostInds = (ages >= ageThreshold & visibility < 0.6) | ...
[tracks(:).consecutiveInvisibleCount]' >= invisibleForTooLong;
% 删除跟踪器列表中对应的跟踪器
tracks = tracks(~lostInds);
end
function displayTrackingResults(frame, tracks)
% 分别绘制每个跟踪器的轨迹和边框
for i = 1:length(tracks)
if tracks(i).age > 1
% 获取跟踪器的中心点和边框
centroid = tracks(i).centroid;
bbox = tracks(i).bbox;
score = tracks(i).score;
% 绘制跟踪器轨迹
plot(centroid(1), centroid(2), 'g.', 'MarkerSize', 10);
text(bbox(1), bbox(2)-20, sprintf('%.1f',score),'BackgroundColor',[.7 .9 .7],'FontSize',6);
if size(bbox,1)==4
bbox = [bbox(1),bbox(2),bbox(3)-bbox(1),bbox(4)-bbox(2)];
end
rectangle('Position', bbox, 'EdgeColor', 'g', 'LineWidth', 2);
end
end
% 显示帧
imshow(frame);
end
```
这段代码实现了一个简单的目标跟踪器,使用序贯航迹关联算法将每个帧中的检测结果与之前跟踪的目标进行匹配。完整的示例代码可以在MathWorks网站上找到。
阅读全文
相关推荐
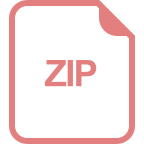
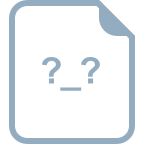
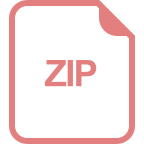



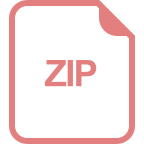
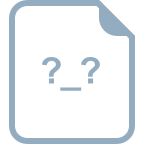
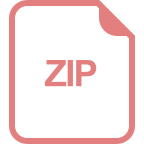
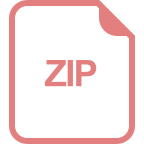
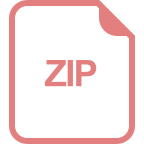
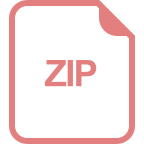
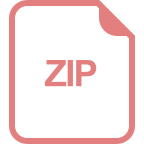
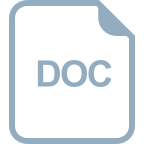
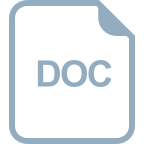

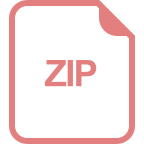