req=urllib.request.Request(url,headers=headers) data=urllib.request.urlopen(req) data=data.read()
时间: 2024-05-25 13:14:18 浏览: 17
这段代码使用 Python 中的 urllib 库发送 HTTP 请求并获取响应数据。
首先,我们需要构造一个 Request 对象,需要传入两个参数:url 表示请求的 URL,headers 是一个字典类型,表示请求头信息。可以通过修改 headers 来模拟浏览器发送请求。
然后使用 urllib.request.urlopen 发送请求并获取响应数据,得到的是一个 file-like object 对象,需要调用 read() 方法获取响应数据的内容。
注意:在 Python 3.x 版本中,urllib.request.urlopen 返回的是 bytes 类型的对象,需要使用 decode() 方法将其转换为字符串类型。
相关问题
request = urllib.request.Request(headers=head,url=url)用的什么请求头
这个代码中的 `headers` 参数指定了一个字典对象 `head`,其中包含了 HTTP 请求的头信息。由于你并没有提供 `head` 的具体内容,因此我无法确定这个请求头的具体内容。
一般来说,HTTP 请求头包含了诸如 User-Agent、Accept、Accept-Language、Referer、Cookie 等信息。其中,User-Agent 是最常用的请求头之一,用于标识客户端的类型和版本信息,以便服务器端根据客户端的不同特性返回不同的内容。不同的 HTTP 客户端(如浏览器、Python 程序等)的 User-Agent 可能会有所不同,因此有些网站可能会根据 User-Agent 来判断客户端的类型和版本,从而返回不同的内容或采取不同的行为。
在 Python 中,通过设置 urllib.request 模块中的 Request 对象的 headers 属性,可以添加自定义的 HTTP 请求头。例如:
``` python
import urllib.request
url = 'https://www.example.com'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36',
'Referer': 'https://www.google.com',
'Cookie': 'foo=bar; baz=qux'
}
req = urllib.request.Request(url, headers=headers)
resp = urllib.request.urlopen(req)
```
在上面的代码中,我们使用了一个自定义的 User-Agent,一个 Referer 和一个 Cookie。这些信息可以通过浏览器的开发者工具或者第三方工具(如 Fiddler)来获取。
import urllib.parse import urllib.request url = 'http://www.qybor.com:8500/shortMessage' #【183.36.116.118】 user_agent = 'Mozilla/4.0 (compatible; MSIE 5.5; Windows NT)' encode = 'UTF-8' username = 'pyhxjnyy' passwd = '03214' needstatus = 'true' phone='17765241887' msg = '您好,您的验证码是:12345【企业宝】' values = { 'username' : username, 'passwd' : passwd, 'needstatus' : needstatus, 'phone' : phone, 'msg' : msg, 'encode' : encode } headers = { 'User-Agent' : user_agent } data = urllib.parse.urlencode(values) req = urllib.request.Request(url+'?'+data) response = urllib.request.urlopen(req) the_page = response.read() print(the_page)
好的,我会帮你调用接口。以下是你提供的代码:
```python
import urllib.parse
import urllib.request
url = 'http://www.qybor.com:8500/shortMessage'
user_agent = 'Mozilla/4.0 (compatible; MSIE 5.5; Windows NT)'
encode = 'UTF-8'
username = 'pyhxjnyy'
passwd = '03214'
needstatus = 'true'
phone = '17765241887'
msg = '您好,您的验证码是:12345【企业宝】'
values = {
'username': username,
'passwd': passwd,
'needstatus': needstatus,
'phone': phone,
'msg': msg,
'encode': encode
}
headers = {'User-Agent': user_agent}
data = urllib.parse.urlencode(values)
req = urllib.request.Request(url + '?' + data, headers=headers)
response = urllib.request.urlopen(req)
the_page = response.read()
print(the_page)
```
请注意,你需要确保你的网络连接正常,并且修改代码中的接口URL、用户名、密码、手机号码以及消息内容等参数,以适应你的实际需求。
相关推荐
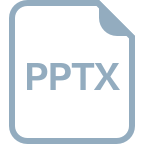
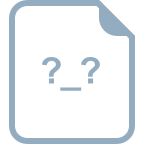
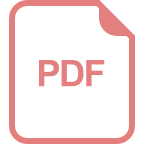










注释以下代码from re import findall from urllib.parse import urljoin from urllib.request import urlopen, Request url = r'http://jwc.sdtbu.edu.cn/info/2002/5418.htm' headers = {'User-Agent':'Mozilla/5.0 (Windows NT 6.1; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/62.0.3202.62 Safari/537.36', 'Referer': url, } # 不加这一项会有防盗链提示 req = Request(url=url, headers=headers) with urlopen(req) as fp: content = fp.read().decode() pattern = r'(.+?)' for fileUrl, fileName in findall(pattern, content): if 'javascript' in fileUrl: continue fileUrl = urljoin(url, fileUrl) req = Request(url=fileUrl, headers=headers) with urlopen(req) as fp1: with open(fileName, 'wb') as fp2: fp2.write(fp1.read())



