C++使用面向对象程序设计方法设计一个程序,实现对复数类型数据进行加、减、乘、除、平方和立方
时间: 2023-12-26 08:04:10 浏览: 73
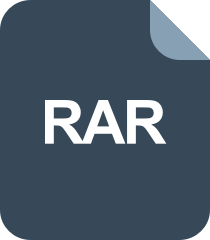
C++面向对象的程序设计
以下是一个简单的复数类型类的实现,包含加、减、乘、除、平方和立方运算:
```cpp
#include <iostream>
#include <cmath>
class Complex {
public:
Complex(double real = 0, double imag = 0) : m_real(real), m_imag(imag) {}
// 加法运算
Complex operator+(const Complex& other) const {
return Complex(m_real + other.m_real, m_imag + other.m_imag);
}
// 减法运算
Complex operator-(const Complex& other) const {
return Complex(m_real - other.m_real, m_imag - other.m_imag);
}
// 乘法运算
Complex operator*(const Complex& other) const {
return Complex(m_real * other.m_real - m_imag * other.m_imag,
m_real * other.m_imag + m_imag * other.m_real);
}
// 除法运算
Complex operator/(const Complex& other) const {
double denominator = other.m_real * other.m_real + other.m_imag * other.m_imag;
return Complex((m_real * other.m_real + m_imag * other.m_imag) / denominator,
(m_imag * other.m_real - m_real * other.m_imag) / denominator);
}
// 平方运算
Complex square() const {
return Complex(m_real * m_real - m_imag * m_imag, 2 * m_real * m_imag);
}
// 立方运算
Complex cube() const {
double real = m_real * m_real - 3 * m_imag * m_imag;
double imag = 3 * m_real * m_imag - m_imag * m_imag;
return Complex(real, imag);
}
// 打印复数
void print() const {
std::cout << m_real << " + " << m_imag << "i" << std::endl;
}
private:
double m_real;
double m_imag;
};
int main() {
Complex c1(1, 2);
Complex c2(3, 4);
// 加法
Complex c3 = c1 + c2;
c3.print(); // 输出 4 + 6i
// 减法
Complex c4 = c1 - c2;
c4.print(); // 输出 -2 - 2i
// 乘法
Complex c5 = c1 * c2;
c5.print(); // 输出 -5 + 10i
// 除法
Complex c6 = c1 / c2;
c6.print(); // 输出 0.44 + 0.08i
// 平方
Complex c7 = c1.square();
c7.print(); // 输出 -3 + 4i
// 立方
Complex c8 = c1.cube();
c8.print(); // 输出 -11 + 2i
return 0;
}
```
需要注意的是,以上实现中,除法运算的分母不能为零。此外,为了避免精度误差,实现中的数学计算应该尽量使用库函数。
阅读全文
相关推荐
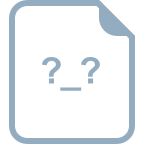
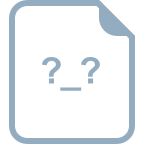



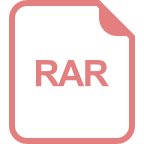
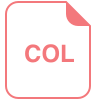




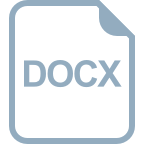
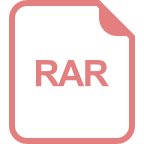
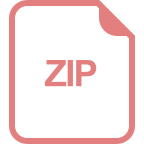
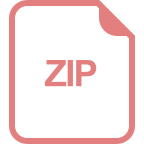
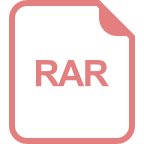