为上述代码添加一个可视化的界面
时间: 2024-02-21 12:59:21 浏览: 67
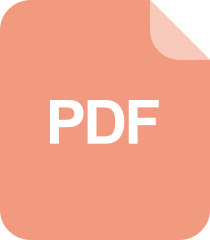
Python Selenium实现无可视化界面过程解析

为上述代码添加一个可视化的界面,可以使用Python的图形界面库Tkinter来实现。以下是一个简单的界面代码示例:
```python
import random
import tkinter as tk
# 定义宠物类
class Pet:
def __init__(self, name, age, hunger, happiness, health):
self.name = name
self.age = age
self.hunger = hunger
self.happiness = happiness
self.health = health
# 宠物吃东西
def eat(self):
self.hunger -= 10
self.happiness += 5
self.health += 5
# 宠物玩耍
def play(self):
self.hunger += 5
self.happiness += 10
self.health += 5
# 宠物看医生
def see_doctor(self):
self.health += 20
# 宠物睡觉
def sleep(self):
self.hunger += 10
self.happiness += 5
self.health += 10
# 宠物打印状态
def print_status(self):
return "名字: {}\n年龄: {}\n饥饿值: {}\n快乐值: {}\n健康值: {}".format(
self.name, self.age, self.hunger, self.happiness, self.health)
# 定义游戏类
class Game:
def __init__(self):
self.pet_list = [] # 存放宠物的列表
self.day = 1 # 游戏进行的天数
# 添加新的宠物
def add_pet(self, name):
age = random.randint(1, 10) # 宠物的年龄随机生成
hunger = random.randint(50, 100) # 宠物的饥饿值随机生成
happiness = random.randint(50, 100) # 宠物的快乐值随机生成
health = random.randint(50, 100) # 宠物的健康值随机生成
pet = Pet(name, age, hunger, happiness, health)
self.pet_list.append(pet)
# 游戏开始
def start(self):
window = tk.Tk()
window.title("电子宠物游戏")
window.geometry("400x400")
def add_pet():
name = name_input.get()
self.add_pet(name)
pet_listbox.insert(tk.END, name)
def select_pet(event):
index = pet_listbox.curselection()[0]
pet = self.pet_list[index]
status_label.config(text=pet.print_status())
def eat():
for pet in self.pet_list:
pet.eat()
def play():
for pet in self.pet_list:
pet.play()
def see_doctor():
for pet in self.pet_list:
pet.see_doctor()
def sleep():
for pet in self.pet_list:
pet.sleep()
name_label = tk.Label(window, text="宠物名字:")
name_label.pack()
name_input = tk.Entry(window)
name_input.pack()
add_pet_button = tk.Button(window, text="添加宠物", command=add_pet)
add_pet_button.pack()
pet_listbox = tk.Listbox(window)
pet_listbox.bind("<<ListboxSelect>>", select_pet)
pet_listbox.pack()
status_label = tk.Label(window, text="")
status_label.pack()
eat_button = tk.Button(window, text="喂食", command=eat)
eat_button.pack()
play_button = tk.Button(window, text="玩耍", command=play)
play_button.pack()
see_doctor_button = tk.Button(window, text="看医生", command=see_doctor)
see_doctor_button.pack()
sleep_button = tk.Button(window, text="睡觉", command=sleep)
sleep_button.pack()
quit_button = tk.Button(window, text="退出游戏", command=window.quit)
quit_button.pack()
window.mainloop()
# 程序入口
if __name__ == "__main__":
game = Game()
game.start()
```
以上代码实现了一个简单的可视化界面,包括添加宠物、选择宠物、查看宠物状态、喂食、玩耍、看医生、睡觉和退出游戏等功能。界面使用了Tkinter库来实现,可以在窗口中显示宠物列表和宠物状态。
阅读全文
相关推荐
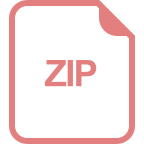
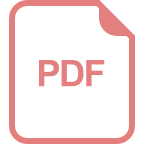















