帮我写这样一段代码:需求 1、使用electron-dl 创建下载下载 2、创建一个队列,用户下载的数据通过一个数组dataArray传递过来,然后循环添加到队列中排队 3、队列拿出数据创建下载器,每次最大创建5个,这五个中只要有其中一个下载结束,那么队列自动弹出一个数据创建一个下载器去下载,直到下载结束。 4、渲染层要渲染:所有下载的数据,有下载进度,有全部暂停、全部取消、暂停后重新开始下载,以及每个下载都有自己的取消、暂停、开始按钮控制。没有进入下载的任务条目在渲染层渲染的进度条是0、 5、进度条使用的是ant design 的progress进度条制作, 6、每次下载结束,就会用 ant-design 的message 弹出提示xxxx(这个数据的名字)+下载完成
时间: 2024-02-25 09:56:19 浏览: 32
好的,这是一个比较复杂的需求,需要涉及到一些electron-dl和队列的操作。我会尽可能详细地给出代码实现,但是可能会有一些需要你自己根据实际情况修改的地方。
首先,需要在渲染进程中创建一个下载队列:
```javascript
const downloadQueue = [];
function addToQueue(item) {
downloadQueue.push(item);
updateDownloadList();
}
function removeFromQueue(item) {
const index = downloadQueue.indexOf(item);
if (index > -1) {
downloadQueue.splice(index, 1);
updateDownloadList();
}
}
function updateDownloadList() {
// 渲染 downloadQueue 中的数据
}
```
然后,在渲染进程中创建一个下载管理器,用于管理下载器的数量和状态:
```javascript
class DownloadManager {
constructor() {
this.maxConcurrentDownloads = 5;
this.activeDownloads = [];
}
async startDownload(item) {
const download = await startDownload(item);
this.activeDownloads.push(download);
this.update();
download.on('done', () => {
this.activeDownloads.splice(this.activeDownloads.indexOf(download), 1);
this.update();
showMessage(`${item.name} 下载完成`);
if (downloadQueue.length > 0) {
this.startDownload(downloadQueue.shift());
}
});
}
pauseAll() {
this.activeDownloads.forEach(download => {
download.pause();
});
this.update();
}
resumeAll() {
this.activeDownloads.forEach(download => {
download.resume();
});
this.update();
}
cancelAll() {
this.activeDownloads.forEach(download => {
download.cancel();
});
this.activeDownloads = [];
this.update();
}
pause(download) {
download.pause();
this.update();
}
resume(download) {
download.resume();
this.update();
}
cancel(download) {
download.cancel();
this.activeDownloads.splice(this.activeDownloads.indexOf(download), 1);
this.update();
}
update() {
// 渲染 activeDownloads 中的数据
}
}
const downloadManager = new DownloadManager();
```
其中,startDownload 方法用于创建一个下载器并开始下载,done 事件用于在下载完成后从 activeDownloads 中删除该下载器并启动下一个任务。pauseAll、resumeAll、cancelAll 方法用于暂停、恢复、取消所有下载任务;pause、resume、cancel 方法用于暂停、恢复、取消单个下载任务。
下面是 startDownload 方法的实现:
```javascript
function startDownload(item) {
return new Promise((resolve, reject) => {
const options = {
directory: item.directory,
filename: item.filename,
onProgress: progress => {
item.progress = progress.percent;
downloadManager.update();
}
};
const download = electronDl(item.url, options);
download.once('done', resolve);
download.once('error', reject);
});
}
```
其中,electronDl 是 electron-dl 库提供的下载函数,options 中包含了下载的目录和文件名,以及进度回调函数 onProgress。
最后是渲染进程中的代码,用于渲染下载列表和控制按钮:
```javascript
function renderDownloadItem(item) {
return `
<div class="download-item">
<div class="download-name">${item.name}</div>
<div class="download-progress">
<Progress percent=${item.progress} />
</div>
<div class="download-controls">
<Button onClick=${() => downloadManager.pause(item)}>暂停</Button>
<Button onClick=${() => downloadManager.resume(item)}>恢复</Button>
<Button onClick=${() => downloadManager.cancel(item)}>取消</Button>
</div>
</div>
`;
}
function renderDownloadList() {
const items = downloadQueue.concat(downloadManager.activeDownloads);
const list = items.map(renderDownloadItem).join('');
// 渲染 list
}
function showMessage(message) {
// 使用 ant-design 的 message 弹出提示消息
}
// 绑定按钮事件
document.querySelector('#pause-all').addEventListener('click', downloadManager.pauseAll.bind(downloadManager));
document.querySelector('#resume-all').addEventListener('click', downloadManager.resumeAll.bind(downloadManager));
document.querySelector('#cancel-all').addEventListener('click', downloadManager.cancelAll.bind(downloadManager));
```
以上代码是一个比较简单的实现,可能还有很多细节需要你自己去完善。希望能够帮到你!
相关推荐
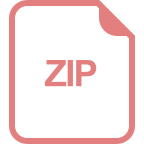
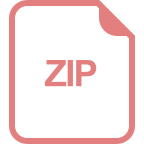
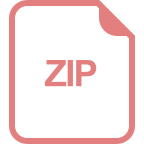














