重庆邮电大学数据结构期末考试题
时间: 2025-01-04 09:36:43 浏览: 8
### 关于重庆邮电大学数据结构课程期末考试题目
对于重庆邮电大学的数据结构课程,其期末考试题目通常会覆盖广泛的知识点,旨在全面评估学生对该学科的理解程度。根据以往的经验和资料[^1],可以推测出该类试题可能涉及的内容范围。
#### 图论部分
图的相关问题是常见考点之一,可能会考察最小生成树算法的应用以及最短路径计算方法。例如:
```python
import heapq
def dijkstra(graph, start):
queue = []
distances = {node: float('infinity') for node in graph}
distances[start] = 0
heapq.heappush(queue, (distances[start], start))
while queue:
current_distance, current_node = heapq.heappop(queue)
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(queue, (distance, neighbor))
return distances
```
此代码实现了Dijkstra单源最短路径算法,在实际考试中,考生不仅需要掌握此类经典算法的具体实现方式,还需要能够灵活运用这些理论解决具体问题。
#### 查找与排序
查找和排序也是重要的考查方向。快速排序作为一种高效的内部排序法经常被提及;而二分查找法则适用于有序数组中的元素定位操作。下面给出了一段简单的Python代码来展示如何利用递归来完成一次完整的快速排序过程:
```python
def quicksort(array):
if len(array) <= 1:
return array
pivot = array[len(array)//2]
left = [x for x in array if x < pivot]
middle = [x for x in array if x == pivot]
right = [x for x in array if x > pivot]
return quicksort(left)+middle+quicksort(right)
```
上述例子展示了基本的编程技巧,但在真实测试环境中还会有更多复杂度更高的变种形式等待解答者去探索。
#### 文件处理
文件读写能力同样是不可忽视的一环。通过编写程序来进行文本解析、CSV表格编辑或是JSON对象转换等活动均有可能成为命题人的关注焦点。这里提供了一个简单的小案例——统计给定目录下的所有`.txt`文档总字数:
```python
from pathlib import Path
total_words_count = sum(len(open(file).read().split())
for file in Path('.').glob('*.txt'))
print(f'Total words count is {total_words_count}')
```
这段脚本遍历当前工作区内的每一个符合条件的目标文件,并累加它们各自含有的单词数量作为最终结果输出显示出来。
---
阅读全文
相关推荐









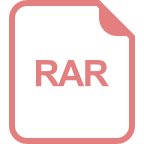





