PCL以二值化描述子为输入的特征匹配代码
时间: 2024-02-13 07:06:38 浏览: 143
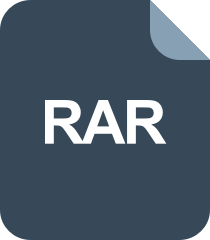
特征匹配源码
以下是使用PCL进行二值化描述子匹配的示例代码:
```cpp
#include <pcl/point_cloud.h>
#include <pcl/point_types.h>
#include <pcl/features/feature.h>
#include <pcl/features/brief.h>
#include <pcl/features/orb.h>
#include <pcl/features/brisk.h>
#include <pcl/registration/correspondence_estimation.h>
#include <pcl/registration/correspondence_rejection.h>
#include <pcl/registration/correspondence_rejection_one_to_one.h>
#include <pcl/registration/correspondence_rejection_median_distance.h>
int main()
{
// 定义两个点云
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud1 (new pcl::PointCloud<pcl::PointXYZ>);
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud2 (new pcl::PointCloud<pcl::PointXYZ>);
// 从文件中读取点云数据
pcl::io::loadPCDFile<pcl::PointXYZ> ("cloud1.pcd", *cloud1);
pcl::io::loadPCDFile<pcl::PointXYZ> ("cloud2.pcd", *cloud2);
// 定义二值化描述子计算对象
pcl::BriefEstimation<pcl::PointXYZ, pcl::Brief> brief;
pcl::OrbEstimation<pcl::PointXYZ, pcl::Orb> orb;
pcl::BriskEstimation<pcl::PointXYZ, pcl::BRISK> brisk;
// 计算两个点云的特征点和二值化描述子
pcl::PointCloud<pcl::Brief>::Ptr descriptors1 (new pcl::PointCloud<pcl::Brief>);
pcl::PointCloud<pcl::Brief>::Ptr descriptors2 (new pcl::PointCloud<pcl::Brief>);
brief.setInputCloud (cloud1);
brief.compute (*descriptors1);
brief.setInputCloud (cloud2);
brief.compute (*descriptors2);
// 定义特征匹配对象
pcl::registration::CorrespondenceEstimation<pcl::Brief, pcl::Brief> est;
est.setInputSource (descriptors1);
est.setInputTarget (descriptors2);
// 计算匹配对
pcl::CorrespondencesPtr correspondences (new pcl::Correspondences);
est.determineCorrespondences (*correspondences);
// 定义匹配对筛选器
pcl::registration::CorrespondenceRejectorOneToOne o2o;
pcl::registration::CorrespondenceRejectorMedianDistance md;
md.setInputCorrespondences (correspondences);
md.getCorrespondences (*correspondences);
o2o.setInputCorrespondences (correspondences);
o2o.getCorrespondences (*correspondences);
// 输出匹配结果
for (const auto& correspondence : *correspondences)
std::cout << correspondence.index_query << " -> " << correspondence.index_match << std::endl;
return 0;
}
```
在上面的代码中,我们使用了PCL中的BriefEstimation、OrbEstimation和BriskEstimation对象来计算二值化描述子,使用CorrespondenceEstimation对象计算匹配对,使用CorrespondenceRejectorOneToOne和CorrespondenceRejectorMedianDistance对象进行筛选。需要注意的是,这里的输入点云类型是PointXYZ,如果需要使用其他类型的点云,需要对BriefEstimation、OrbEstimation和BriskEstimation对象进行相应的修改。
阅读全文
相关推荐
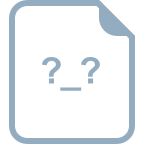
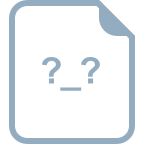



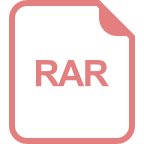
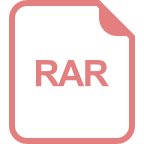
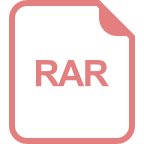
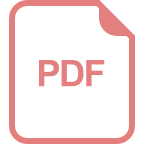
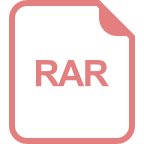
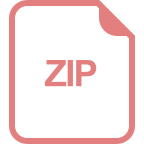
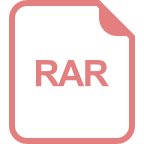
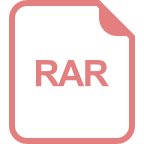
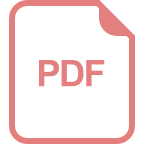
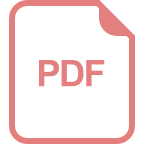