void saveItemsToFile(const string& filename) { ofstream outFile(filename); //默认以输出方式打开文件 if (outFile.is_open()) { for (Item* item : items) { if (Book* book = dynamic_cast<Book*>(item)) { outFile << book->ID << "," << book->title << "," << book->author << "," << book->rating << "," << book->publisher << "," << book->isbn << "," << book->pages << ",book" << endl; } else if (VideoCD* videoCD = dynamic_cast<VideoCD*>(item)) { outFile << videoCD->ID << "," << videoCD->title << "," << videoCD->author << "," << videoCD->rating << "," << videoCD->producer << "," << videoCD->releaseYear << ",videocd" << endl; } else if (Picture* picture = dynamic_cast<Picture*>(item)) { outFile << picture->ID << "," << picture->title << "," << picture->author << "," << picture->rating << "," << picture->country << "," << picture->width << "," << picture->height << ",picture" << endl; } } outFile.close(); cout << "物品信息已成功存入文件!" << endl; } else { cout << "无法打开文件!" << endl; } }
时间: 2024-04-14 22:04:45 浏览: 35
这是一个保存物品信息到文件的函数,它接受一个文件名作为参数。函数首先创建一个 ofstream 对象 outFile 并用默认的输出方式打开指定文件。接着,函数遍历一个名为 items 的容器,这个容器中包含了多种类型的物品,如书籍、视频光盘和图片。对于每个物品,函数使用 dynamic_cast 将其转换为对应的类型,然后将物品的各项信息写入 outFile 中。最后,函数关闭 outFile 并输出一条保存成功的信息,或者在无法打开文件时输出一条错误信息。
相关问题
void writeToFile(const vector<Student>& students, const string& fileName) { ofstream outFile(fileName); for (const auto& s : students) { outFile << s.name << endl; for (const auto& c : s.courses) { outFile << c.name << " " << c.credit << " " << c.term << " " << c.score << endl; } outFile << endl; } outFile.close(); }如何添加文件路径
要添加文件路径,只需要在文件名前面加上路径即可。例如,如果你要将文件保存在名为 "data" 的文件夹下,可以这样修改代码:
```c++
void writeToFile(const vector<Student>& students, const string& fileName) {
ofstream outFile("data/" + fileName); // 添加文件路径
for (const auto& s : students) {
outFile << s.name << endl;
for (const auto& c : s.courses) {
outFile << c.name << " " << c.credit << " " << c.term << " " << c.score << endl;
}
outFile << endl;
}
outFile.close();
}
```
这样,文件就会被保存在 "data" 文件夹下了。当然,如果你要保存到其他路径,只需要修改路径即可。注意,如果路径不存在,程序会报错,所以你需要先创建好需要保存的文件夹。
[Error] no matching function for call to 'std:basic_ofstream< char>:basic_ofstream(const string&)'
这个错误是由于在创建 `std::ofstream` 对象时传递的参数类型不正确导致的。`std::ofstream` 构造函数需要一个 `const char*` 类型的参数,而你传递的参数是一个 `std::string` 类型。
你可以将 `std::string` 类型的参数转换成 `const char*` 类型,有两种方法可以实现:
1. 使用 `std::string` 对象的 `c_str()` 函数,它返回一个指向字符串的 `const char*` 指针。例如:
```
std::string filename = "file.txt";
std::ofstream outfile(filename.c_str());
```
2. 使用 `std::string` 类型的构造函数,它接受一个 `const char*` 类型的参数。例如:
```
std::string filename = "file.txt";
std::ofstream outfile(filename.data());
```
这两种方法都可以解决这个错误。希望能帮到你!
相关推荐
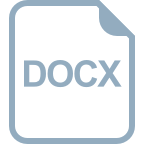
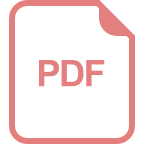
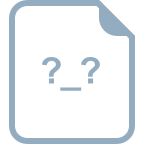












